mirror of
https://github.com/ruby/ruby.git
synced 2022-11-09 12:17:21 -05:00
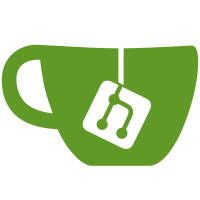
Methods with optional parameters don't always start executing at the first PC, but we compile all methods assuming that they do. This commit adds a guard to ensure that we're actually starting at the first PC for methods with optional params
50 lines
1.1 KiB
C
50 lines
1.1 KiB
C
#ifndef YJIT_CODEGEN_H
|
|
#define YJIT_CODEGEN_H 1
|
|
|
|
#include "stddef.h"
|
|
#include "yjit_core.h"
|
|
|
|
// Code blocks we generate code into
|
|
extern codeblock_t *cb;
|
|
extern codeblock_t *ocb;
|
|
|
|
// Code generation state
|
|
typedef struct JITState
|
|
{
|
|
// Block version being compiled
|
|
block_t* block;
|
|
|
|
// Instruction sequence this is associated with
|
|
const rb_iseq_t *iseq;
|
|
|
|
// Index of the current instruction being compiled
|
|
uint32_t insn_idx;
|
|
|
|
// Opcode for the instruction being compiled
|
|
int opcode;
|
|
|
|
// PC of the instruction being compiled
|
|
VALUE *pc;
|
|
|
|
// Execution context when compilation started
|
|
// This allows us to peek at run-time values
|
|
rb_execution_context_t* ec;
|
|
|
|
} jitstate_t;
|
|
|
|
typedef enum codegen_status {
|
|
YJIT_END_BLOCK,
|
|
YJIT_KEEP_COMPILING,
|
|
YJIT_CANT_COMPILE
|
|
} codegen_status_t;
|
|
|
|
// Code generation function signature
|
|
typedef codegen_status_t (*codegen_fn)(jitstate_t* jit, ctx_t* ctx);
|
|
|
|
uint8_t* yjit_entry_prologue(const rb_iseq_t* iseq);
|
|
|
|
void yjit_gen_block(block_t* block, rb_execution_context_t* ec);
|
|
|
|
void yjit_init_codegen(void);
|
|
|
|
#endif // #ifndef YJIT_CODEGEN_H
|