mirror of
https://github.com/sinatra/sinatra
synced 2023-03-27 23:18:01 -04:00
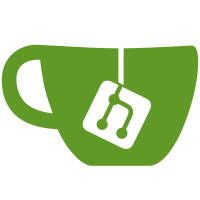
* Initialize rubocop * Style/StringLiterals: prefer single quotes * Style/AndOr: use `&&` and `||`, instead of `and` and `or` * Style/HashSyntax: use new hash syntax * Layout/EmptyLineAfterGuardClause: add empty lines after guard clause * Style/SingleLineMethods: temporary disable It breaks layout of the code, it is better to fix it manually * Style/Proc: prefer `proc` vs `Proc.new` * Disable Lint/AmbiguousBlockAssociation It affects proc definitions for sinatra DSL * Disable Style/CaseEquality * Lint/UnusedBlockArgument: put underscore in front of it * Style/Alias: prefer alias vs alias_method in a class body * Layout/EmptyLineBetweenDefs: add empty lines between defs * Style/ParallelAssignment: don't use parallel assigment * Style/RegexpLiteral: prefer %r for regular expressions * Naming/UncommunicativeMethodParamName: fix abbrevs * Style/PerlBackrefs: disable cop * Layout/SpaceAfterComma: add missing spaces * Style/Documentation: disable cop * Style/FrozenStringLiteralComment: add frozen_string_literal * Layout/AlignHash: align hash * Layout/ExtraSpacing: allow for alignment * Layout/SpaceAroundOperators: add missing spaces * Style/Not: prefer `!` instead of `not` * Style/GuardClause: add guard conditions * Style/MutableConstant: freeze contants * Lint/IneffectiveAccessModifier: disable cop * Lint/RescueException: disable cop * Style/SpecialGlobalVars: disable cop * Layout/DotPosition: fix position of dot for multiline method chains * Layout/SpaceInsideArrayLiteralBrackets: don't use spaces inside arrays * Layout/SpaceInsideBlockBraces: add space for blocks * Layout/SpaceInsideHashLiteralBraces: add spaces for hashes * Style/FormatString: use format string syntax * Style/StderrPuts: `warn` is preferable to `$stderr.puts` * Bundler/DuplicatedGem: disable cop * Layout/AlignArray: fix warning * Lint/AssignmentInCondition: remove assignments from conditions * Layout/IndentHeredoc: disable cop * Layout/SpaceInsideParens: remove extra spaces * Lint/UnusedMethodArgument: put underscore in front of unused arg * Naming/RescuedExceptionsVariableName: use `e` for exceptions * Style/CommentedKeyword: put comments before the method * Style/FormatStringToken: disable cop * Style/MultilineIfModifier: move condition before the method * Style/SignalException: prefer `raise` to `fail` * Style/SymbolArray: prefer %i for array of symbols * Gemspec/OrderedDependencies: Use alphabetical order for dependencies * Lint/UselessAccessModifier: disable cop * Naming/HeredocDelimiterNaming: change delimiter's name * Style/ClassCheck: prefer `is_a?` to `kind_of?` * Style/ClassVars: disable cop * Style/Encoding: remove coding comment * Style/RedundantParentheses: remove extra parentheses * Style/StringLiteralsInInterpolation: prefer singl quotes * Layout/AlignArguments: fix alignment * Layout/ClosingHeredocIndentation: align heredoc * Layout/EmptyLineAfterMagicComment: add empty line * Set RubyVersion for rubocop * Lint/UselessAssignment: disable cop * Style/EmptyLiteral: disable cop Causes test failures * Minor code-style fixes with --safe-auto-correct option * Disable the rest of the cops that cause warnings It would be easier to re-enable them in separate PRs * Add rubocop check to the default Rake task * Update to rubocop 1.32.0 * Rubocop updates for rack-protection and sinatra-contrib * Disable Style/SlicingWithRange cop * Make suggested updates Co-authored-by: Jordan Owens <jkowens@gmail.com>
77 lines
2.1 KiB
Ruby
77 lines
2.1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require 'rack/protection'
|
|
require 'pathname'
|
|
|
|
module Rack
|
|
module Protection
|
|
##
|
|
# Prevented attack:: Cookie Tossing
|
|
# Supported browsers:: all
|
|
# More infos:: https://github.com/blog/1466-yummy-cookies-across-domains
|
|
#
|
|
# Does not accept HTTP requests if the HTTP_COOKIE header contains more than one
|
|
# session cookie. This does not protect against a cookie overflow attack.
|
|
#
|
|
# Options:
|
|
#
|
|
# session_key:: The name of the session cookie (default: 'rack.session')
|
|
class CookieTossing < Base
|
|
default_reaction :deny
|
|
|
|
def call(env)
|
|
status, headers, body = super
|
|
response = Rack::Response.new(body, status, headers)
|
|
request = Rack::Request.new(env)
|
|
remove_bad_cookies(request, response)
|
|
response.finish
|
|
end
|
|
|
|
def accepts?(env)
|
|
cookie_header = env['HTTP_COOKIE']
|
|
cookies = Rack::Utils.parse_query(cookie_header, ';,') { |s| s }
|
|
cookies.each do |k, v|
|
|
if (k == session_key && Array(v).size > 1) ||
|
|
(k != session_key && Rack::Utils.unescape(k) == session_key)
|
|
bad_cookies << k
|
|
end
|
|
end
|
|
bad_cookies.empty?
|
|
end
|
|
|
|
def remove_bad_cookies(request, response)
|
|
return if bad_cookies.empty?
|
|
|
|
paths = cookie_paths(request.path)
|
|
bad_cookies.each do |name|
|
|
paths.each { |path| response.set_cookie name, empty_cookie(request.host, path) }
|
|
end
|
|
end
|
|
|
|
def redirect(env)
|
|
request = Request.new(env)
|
|
warn env, "attack prevented by #{self.class}"
|
|
[302, { 'Content-Type' => 'text/html', 'Location' => request.path }, []]
|
|
end
|
|
|
|
def bad_cookies
|
|
@bad_cookies ||= []
|
|
end
|
|
|
|
def cookie_paths(path)
|
|
path = '/' if path.to_s.empty?
|
|
paths = []
|
|
Pathname.new(path).descend { |p| paths << p.to_s }
|
|
paths
|
|
end
|
|
|
|
def empty_cookie(host, path)
|
|
{ value: '', domain: host, path: path, expires: Time.at(0) }
|
|
end
|
|
|
|
def session_key
|
|
@session_key ||= options[:session_key]
|
|
end
|
|
end
|
|
end
|
|
end
|