mirror of
https://github.com/sinatra/sinatra
synced 2023-03-27 23:18:01 -04:00
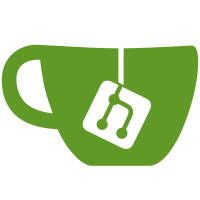
We use minitest for Sinatra's test suite but we weren't using its rake task. I've updated the Rakefile to require and use Minitest default rake task to simplify. Another change is to rename the `helper.rb` file to `test_helper.rb` because I think that name is used more in the community and require it directly without calling `File.expand_path`
40 lines
1 KiB
Ruby
40 lines
1 KiB
Ruby
require_relative 'test_helper'
|
|
require File.expand_path('integration_async_helper', __dir__)
|
|
|
|
# These tests are like integration_test, but they test asynchronous streaming.
|
|
class IntegrationAsyncTest < Minitest::Test
|
|
extend IntegrationAsyncHelper
|
|
attr_accessor :server
|
|
|
|
it 'streams async' do
|
|
Timeout.timeout(3) do
|
|
chunks = []
|
|
server.get_stream '/async' do |chunk|
|
|
next if chunk.empty?
|
|
chunks << chunk
|
|
case chunk
|
|
when "hi!" then server.get "/send?msg=hello"
|
|
when "hello" then server.get "/send?close=1"
|
|
end
|
|
end
|
|
|
|
assert_equal ['hi!', 'hello'], chunks
|
|
end
|
|
end
|
|
|
|
it 'streams async from subclass' do
|
|
Timeout.timeout(3) do
|
|
chunks = []
|
|
server.get_stream '/subclass/async' do |chunk|
|
|
next if chunk.empty?
|
|
chunks << chunk
|
|
case chunk
|
|
when "hi!" then server.get "/subclass/send?msg=hello"
|
|
when "hello" then server.get "/subclass/send?close=1"
|
|
end
|
|
end
|
|
|
|
assert_equal ['hi!', 'hello'], chunks
|
|
end
|
|
end
|
|
end
|