mirror of
https://github.com/sinatra/sinatra
synced 2023-03-27 23:18:01 -04:00
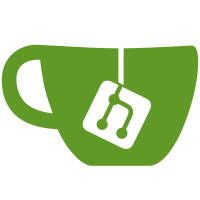
The "spec" task has been removed and Rake's built in test helper is used to run specs now so we should be able to test with multiple installed versions of Ruby.
58 lines
1.3 KiB
Ruby
58 lines
1.3 KiB
Ruby
require File.dirname(__FILE__) + '/helper'
|
|
|
|
describe "Middleware" do
|
|
before do
|
|
@app = mock_app(Sinatra::Default) {
|
|
get '/*' do
|
|
response.headers['X-Tests'] = env['test.ran'].join(', ')
|
|
env['PATH_INFO']
|
|
end
|
|
}
|
|
end
|
|
|
|
class MockMiddleware < Struct.new(:app)
|
|
def call(env)
|
|
(env['test.ran'] ||= []) << self.class.to_s
|
|
app.call(env)
|
|
end
|
|
end
|
|
|
|
class UpcaseMiddleware < MockMiddleware
|
|
def call(env)
|
|
env['PATH_INFO'] = env['PATH_INFO'].upcase
|
|
super
|
|
end
|
|
end
|
|
|
|
it "is added with Sinatra::Application.use" do
|
|
@app.use UpcaseMiddleware
|
|
get '/hello-world'
|
|
assert ok?
|
|
assert_equal '/HELLO-WORLD', body
|
|
end
|
|
|
|
class DowncaseMiddleware < MockMiddleware
|
|
def call(env)
|
|
env['PATH_INFO'] = env['PATH_INFO'].downcase
|
|
super
|
|
end
|
|
end
|
|
|
|
it "runs in the order defined" do
|
|
@app.use UpcaseMiddleware
|
|
@app.use DowncaseMiddleware
|
|
get '/Foo'
|
|
assert_equal "/foo", body
|
|
assert_equal "UpcaseMiddleware, DowncaseMiddleware", response['X-Tests']
|
|
end
|
|
|
|
it "resets the prebuilt pipeline when new middleware is added" do
|
|
@app.use UpcaseMiddleware
|
|
get '/Foo'
|
|
assert_equal "/FOO", body
|
|
@app.use DowncaseMiddleware
|
|
get '/Foo'
|
|
assert_equal '/foo', body
|
|
assert_equal "UpcaseMiddleware, DowncaseMiddleware", response['X-Tests']
|
|
end
|
|
end
|