mirror of
https://github.com/sinatra/sinatra
synced 2023-03-27 23:18:01 -04:00
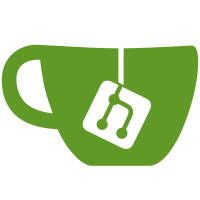
This moves the :halt catch out so that all routing code runs within one giant catch block instead of running each type of handler in its own catch block. This required some cleanup in the error handling code, which cleaned things up quite a bit. This corrects two issues: 1. halt with > 1 args causes ArgumentError http://sinatra.lighthouseapp.com/projects/9779/tickets/131 2. halting in a before filter doesn't modify response http://sinatra.lighthouseapp.com/projects/9779/tickets/127 We still need to split up the more epic methods (#route!, #invoke) but the logic is pretty sound at this point.
99 lines
1.8 KiB
Ruby
99 lines
1.8 KiB
Ruby
require File.dirname(__FILE__) + '/helper'
|
|
|
|
describe "Filters" do
|
|
it "executes filters in the order defined" do
|
|
count = 0
|
|
mock_app do
|
|
get('/') { 'Hello World' }
|
|
before {
|
|
assert_equal 0, count
|
|
count = 1
|
|
}
|
|
before {
|
|
assert_equal 1, count
|
|
count = 2
|
|
}
|
|
end
|
|
|
|
get '/'
|
|
assert ok?
|
|
assert_equal 2, count
|
|
assert_equal 'Hello World', body
|
|
end
|
|
|
|
it "allows filters to modify the request" do
|
|
mock_app {
|
|
get('/foo') { 'foo' }
|
|
get('/bar') { 'bar' }
|
|
before { request.path_info = '/bar' }
|
|
}
|
|
|
|
get '/foo'
|
|
assert ok?
|
|
assert_equal 'bar', body
|
|
end
|
|
|
|
it "can modify instance variables available to routes" do
|
|
mock_app {
|
|
before { @foo = 'bar' }
|
|
get('/foo') { @foo }
|
|
}
|
|
|
|
get '/foo'
|
|
assert ok?
|
|
assert_equal 'bar', body
|
|
end
|
|
|
|
it "allows redirects in filters" do
|
|
mock_app {
|
|
before { redirect '/bar' }
|
|
get('/foo') do
|
|
fail 'before block should have halted processing'
|
|
'ORLY?!'
|
|
end
|
|
}
|
|
|
|
get '/foo'
|
|
assert redirect?
|
|
assert_equal '/bar', response['Location']
|
|
assert_equal '', body
|
|
end
|
|
|
|
it "does not modify the response with its return value" do
|
|
mock_app {
|
|
before { 'Hello World!' }
|
|
get '/foo' do
|
|
assert_equal [], response.body
|
|
'cool'
|
|
end
|
|
}
|
|
|
|
get '/foo'
|
|
assert ok?
|
|
assert_equal 'cool', body
|
|
end
|
|
|
|
it "does modify the response with halt" do
|
|
mock_app {
|
|
before { halt 302, 'Hi' }
|
|
get '/foo' do
|
|
"should not happen"
|
|
end
|
|
}
|
|
|
|
get '/foo'
|
|
assert_equal 302, response.status
|
|
assert_equal 'Hi', body
|
|
end
|
|
|
|
it "gives you access to params" do
|
|
mock_app {
|
|
before { @foo = params['foo'] }
|
|
get('/foo') { @foo }
|
|
}
|
|
|
|
get '/foo?foo=cool'
|
|
assert ok?
|
|
assert_equal 'cool', body
|
|
end
|
|
end
|