mirror of
https://github.com/teamcapybara/capybara.git
synced 2022-11-09 12:08:07 -05:00
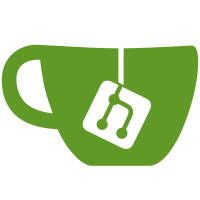
With browser-based drivers, the has_value?, has_select?, and has_checked_field? matchers (and their negative counterparts) did not always work correctly. It is not possible to determine the dynamic state of inputs purely with XPath, because XPath has access only to the value, selected, and checked _attributes_, and only the corresponding _properties_ change in response to user input. This commit replaces the pure XPath implementation with one that retrieves all matching inputs and then filters the results using driver-specific means that do reflect user input.
74 lines
1.3 KiB
Ruby
74 lines
1.3 KiB
Ruby
module Capybara
|
|
module Driver
|
|
class Node
|
|
attr_reader :driver, :native
|
|
|
|
def initialize(driver, native)
|
|
@driver = driver
|
|
@native = native
|
|
end
|
|
|
|
def text
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def [](name)
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def value
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def set(value)
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def select_option
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def unselect_option
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def click
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def drag_to(element)
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def tag_name
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def visible?
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def checked?
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def selected?
|
|
raise NotImplementedError
|
|
end
|
|
|
|
def path
|
|
raise NotSupportedByDriverError
|
|
end
|
|
|
|
def trigger(event)
|
|
raise NotSupportedByDriverError
|
|
end
|
|
|
|
def inspect
|
|
%(#<Capybara::Driver::Node tag="#{tag_name}" path="#{path}">)
|
|
rescue NotSupportedByDriverError
|
|
%(#<Capybara::Driver::Node tag="#{tag_name}">)
|
|
end
|
|
end
|
|
end
|
|
end
|