mirror of
https://github.com/teamcapybara/capybara.git
synced 2022-11-09 12:08:07 -05:00
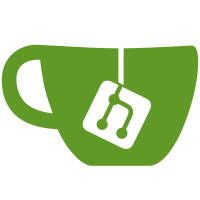
With browser-based drivers, the has_value?, has_select?, and has_checked_field? matchers (and their negative counterparts) did not always work correctly. It is not possible to determine the dynamic state of inputs purely with XPath, because XPath has access only to the value, selected, and checked _attributes_, and only the corresponding _properties_ change in response to user input. This commit replaces the pure XPath implementation with one that retrieves all matching inputs and then filters the results using driver-specific means that do reflect user input.
129 lines
5.4 KiB
Ruby
129 lines
5.4 KiB
Ruby
shared_examples_for "has_select" do
|
|
describe '#has_select?' do
|
|
before { @session.visit('/form') }
|
|
|
|
it "should be true if the field is on the page" do
|
|
@session.should have_select('Locale')
|
|
@session.should have_select('form_region')
|
|
@session.should have_select('Languages')
|
|
end
|
|
|
|
it "should be false if the field is not on the page" do
|
|
@session.should_not have_select('Monkey')
|
|
end
|
|
|
|
context 'with selected value' do
|
|
it "should be true if a field with the given value is on the page" do
|
|
@session.should have_select('form_locale', :selected => 'English')
|
|
@session.should have_select('Region', :selected => 'Norway')
|
|
@session.should have_select('Underwear', :selected => ['Briefs', 'Commando'])
|
|
end
|
|
|
|
it "should be false if the given field is not on the page" do
|
|
@session.should_not have_select('Locale', :selected => 'Swedish')
|
|
@session.should_not have_select('Does not exist', :selected => 'John')
|
|
@session.should_not have_select('City', :selected => 'Not there')
|
|
@session.should_not have_select('Underwear', :selected => ['Briefs', 'Nonexistant'])
|
|
@session.should_not have_select('Underwear', :selected => ['Briefs', 'Boxers'])
|
|
end
|
|
|
|
it "should be true after the given value is selected" do
|
|
@session.select('Swedish', :from => 'Locale')
|
|
@session.should have_select('Locale', :selected => 'Swedish')
|
|
end
|
|
|
|
it "should be false after a different value is selected" do
|
|
@session.select('Swedish', :from => 'Locale')
|
|
@session.should_not have_select('Locale', :selected => 'English')
|
|
end
|
|
|
|
it "should be true after the given values are selected" do
|
|
@session.select('Boxers', :from => 'Underwear')
|
|
@session.should have_select('Underwear', :selected => ['Briefs', 'Boxers', 'Commando'])
|
|
end
|
|
|
|
it "should be false after one of the values is unselected" do
|
|
@session.unselect('Briefs', :from => 'Underwear')
|
|
@session.should_not have_select('Underwear', :selected => ['Briefs', 'Commando'])
|
|
end
|
|
end
|
|
|
|
context 'with options' do
|
|
it "should be true if a field with the given options is on the page" do
|
|
@session.should have_select('form_locale', :options => ['English'])
|
|
@session.should have_select('Region', :options => ['Norway', 'Sweden'])
|
|
end
|
|
|
|
it "should be false if the given field is not on the page" do
|
|
@session.should_not have_select('Locale', :options => ['Not there'])
|
|
@session.should_not have_select('Does not exist', :options => ['John'])
|
|
@session.should_not have_select('City', :options => ['London', 'Made up city'])
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#has_no_select?' do
|
|
before { @session.visit('/form') }
|
|
|
|
it "should be false if the field is on the page" do
|
|
@session.should_not have_no_select('Locale')
|
|
@session.should_not have_no_select('form_region')
|
|
@session.should_not have_no_select('Languages')
|
|
end
|
|
|
|
it "should be true if the field is not on the page" do
|
|
@session.should have_no_select('Monkey')
|
|
end
|
|
|
|
context 'with selected value' do
|
|
it "should be false if a field with the given value is on the page" do
|
|
@session.should_not have_no_select('form_locale', :selected => 'English')
|
|
@session.should_not have_no_select('Region', :selected => 'Norway')
|
|
@session.should_not have_no_select('Underwear', :selected => ['Briefs', 'Commando'])
|
|
end
|
|
|
|
it "should be true if the given field is not on the page" do
|
|
@session.should have_no_select('Locale', :selected => 'Swedish')
|
|
@session.should have_no_select('Does not exist', :selected => 'John')
|
|
@session.should have_no_select('City', :selected => 'Not there')
|
|
@session.should have_no_select('Underwear', :selected => ['Briefs', 'Nonexistant'])
|
|
@session.should have_no_select('Underwear', :selected => ['Briefs', 'Boxers'])
|
|
end
|
|
|
|
it "should be false after the given value is selected" do
|
|
@session.select('Swedish', :from => 'Locale')
|
|
@session.should_not have_no_select('Locale', :selected => 'Swedish')
|
|
end
|
|
|
|
it "should be true after a different value is selected" do
|
|
@session.select('Swedish', :from => 'Locale')
|
|
@session.should have_no_select('Locale', :selected => 'English')
|
|
end
|
|
|
|
it "should be false after the given values are selected" do
|
|
@session.select('Boxers', :from => 'Underwear')
|
|
@session.should_not have_no_select('Underwear', :selected => ['Briefs', 'Boxers', 'Commando'])
|
|
end
|
|
|
|
it "should be true after one of the values is unselected" do
|
|
@session.unselect('Briefs', :from => 'Underwear')
|
|
@session.should have_no_select('Underwear', :selected => ['Briefs', 'Commando'])
|
|
end
|
|
end
|
|
|
|
context 'with options' do
|
|
it "should be false if a field with the given options is on the page" do
|
|
@session.should_not have_no_select('form_locale', :options => ['English'])
|
|
@session.should_not have_no_select('Region', :options => ['Norway', 'Sweden'])
|
|
end
|
|
|
|
it "should be true if the given field is not on the page" do
|
|
@session.should have_no_select('Locale', :options => ['Not there'])
|
|
@session.should have_no_select('Does not exist', :options => ['John'])
|
|
@session.should have_no_select('City', :options => ['London', 'Made up city'])
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
|