mirror of
https://github.com/teampoltergeist/poltergeist.git
synced 2022-11-09 12:05:00 -05:00
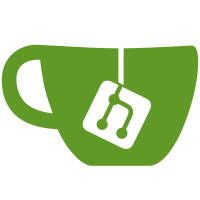
Ask, don't tell, or something like that! Now the WebSocketServer decides what port it will use and everything else relies on that. By default an ephemeral port is used, but a fixed port number can be passed in too. This work is necessary to support Windows (#240) because on Windows a TCP socket cannot be closed and immediately reopened, which we are doing in the tests. So this change means that when restarting, a new ephemeral port can be used by the server.
93 lines
2.8 KiB
Ruby
93 lines
2.8 KiB
Ruby
require 'spec_helper'
|
|
|
|
module Capybara::Poltergeist
|
|
describe Client do
|
|
let(:server) { stub(port: 6000) }
|
|
|
|
subject { Client.new(server) }
|
|
|
|
it 'raises an error if phantomjs is too old' do
|
|
`true` # stubbing $?
|
|
subject.stub(:`).with('phantomjs --version').and_return("1.3.0\n")
|
|
expect { subject.start }.to raise_error(PhantomJSTooOld)
|
|
end
|
|
|
|
it 'shows the detected version in the version error message' do
|
|
`true` # stubbing $?
|
|
subject.stub(:`).with('phantomjs --version').and_return("1.3.0\n")
|
|
begin
|
|
subject.start
|
|
rescue PhantomJSTooOld => e
|
|
e.message.should include('1.3.0')
|
|
end
|
|
end
|
|
|
|
it 'raises an error if phantomjs returns a non-zero exit code' do
|
|
subject = Client.new(server, :path => 'exit 42 && ')
|
|
expect { subject.start }.to raise_error(Error)
|
|
|
|
begin
|
|
subject.start
|
|
rescue PhantomJSFailed => e
|
|
e.message.should include('42')
|
|
end
|
|
end
|
|
|
|
context 'with width and height specified' do
|
|
subject { Client.new(server, :window_size => [800, 600]) }
|
|
|
|
it 'starts phantomjs, passing the width and height through' do
|
|
Process.should_receive(:spawn).with("phantomjs", Client::PHANTOMJS_SCRIPT, "6000", "800", "600", out: $stdout)
|
|
subject.start
|
|
end
|
|
end
|
|
|
|
context 'with a custom phantomjs_logger' do
|
|
subject { Client.new(server, :phantomjs_logger => :my_custom_logger, :window_size => [800, 600]) }
|
|
|
|
it 'starts phantomjs, capturing the STDOUT to custom phantomjs_logger' do
|
|
Process.should_receive(:spawn).with("phantomjs", Client::PHANTOMJS_SCRIPT, "6000", "800", "600", out: :my_custom_logger)
|
|
subject.start
|
|
end
|
|
end
|
|
|
|
context 'with additional command-line options' do
|
|
subject { Client.new(server, :phantomjs_options => %w[--ignore-ssl-error=yes --load-images=no]) }
|
|
|
|
it 'passed additional command-line options to phantomjs' do
|
|
Process.should_receive(:spawn).with("phantomjs", '--ignore-ssl-error=yes', '--load-images=no', anything, anything, anything, anything, out: $stdout)
|
|
subject.start
|
|
end
|
|
end
|
|
|
|
it "forcibly kills the child if it doesn't respond to SIGTERM" do
|
|
begin
|
|
class << Process
|
|
alias old_wait wait
|
|
end
|
|
|
|
client = Client.new(server)
|
|
Process.stub(spawn: 5678)
|
|
client.start
|
|
|
|
Process.should_receive(:kill).with('TERM', 5678).ordered
|
|
|
|
count = 0
|
|
Process.singleton_class.send(:define_method, :wait) do |*args|
|
|
count += 1
|
|
count == 1 ? sleep(3) : 0
|
|
end
|
|
|
|
Process.should_receive(:kill).with('KILL', 5678).ordered
|
|
|
|
client.stop
|
|
ensure
|
|
class << Process
|
|
undef wait
|
|
alias wait old_wait
|
|
undef old_wait
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|