mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
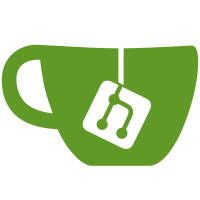
* Fix typo "delcaration" -> "declaration" * Fix typo "Ususually" -> "Usually" * Fix typo "assocition" -> "association" * Fix typo "vaue" -> "value"
65 lines
1.8 KiB
Ruby
65 lines
1.8 KiB
Ruby
describe FactoryBot::Attribute::Dynamic do
|
|
let(:name) { :first_name }
|
|
let(:block) { -> {} }
|
|
|
|
subject { FactoryBot::Attribute::Dynamic.new(name, false, block) }
|
|
|
|
its(:name) { should eq name }
|
|
|
|
context "with a block returning a static value" do
|
|
let(:block) { -> { "value" } }
|
|
|
|
it "returns the value when executing the proc" do
|
|
expect(subject.to_proc.call).to eq "value"
|
|
end
|
|
end
|
|
|
|
context "with a block returning its block-level variable" do
|
|
let(:block) { ->(thing) { thing } }
|
|
|
|
it "returns self when executing the proc" do
|
|
expect(subject.to_proc.call).to eq subject
|
|
end
|
|
end
|
|
|
|
context "with a block referencing an attribute on the attribute" do
|
|
let(:block) { -> { attribute_defined_on_attribute } }
|
|
let(:result) { "other attribute value" }
|
|
|
|
before do
|
|
# Define an '#attribute_defined_on_attribute' instance method allowing it
|
|
# be mocked. Usually this is determined via '#method_missing'
|
|
missing_methods = Module.new {
|
|
def attribute_defined_on_attribute(*args)
|
|
end
|
|
}
|
|
subject.extend(missing_methods)
|
|
|
|
allow(subject)
|
|
.to receive(:attribute_defined_on_attribute).and_return result
|
|
end
|
|
|
|
it "evaluates the attribute from the attribute" do
|
|
expect(subject.to_proc.call).to eq result
|
|
end
|
|
end
|
|
|
|
context "with a block returning a sequence" do
|
|
let(:block) do
|
|
-> do
|
|
FactoryBot::Internal.register_sequence(
|
|
FactoryBot::Sequence.new(:email, 1) { |n| "foo#{n}" }
|
|
)
|
|
end
|
|
end
|
|
|
|
it "raises a sequence abuse error" do
|
|
expect { subject.to_proc.call }.to raise_error(FactoryBot::SequenceAbuseError)
|
|
end
|
|
end
|
|
end
|
|
|
|
describe FactoryBot::Attribute::Dynamic, "with a string name" do
|
|
subject { FactoryBot::Attribute::Dynamic.new("name", false, -> {}) }
|
|
its(:name) { should eq :name }
|
|
end
|