mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
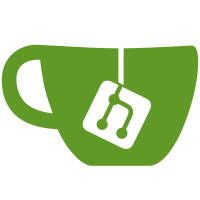
This runs all of the automatic cops that came in since rubocop 0.68. None of these changes were manual.
60 lines
1.3 KiB
Ruby
60 lines
1.3 KiB
Ruby
describe "attribute overrides" do
|
|
before do
|
|
define_model("User", admin: :boolean)
|
|
define_model("Post", title: :string,
|
|
secure: :boolean,
|
|
user_id: :integer) do
|
|
belongs_to :user
|
|
|
|
def secure=(value)
|
|
return unless user&.admin?
|
|
|
|
write_attribute(:secure, value)
|
|
end
|
|
end
|
|
|
|
FactoryBot.define do
|
|
factory :user do
|
|
factory :admin do
|
|
admin { true }
|
|
end
|
|
end
|
|
|
|
factory :post do
|
|
user
|
|
title { "default title" }
|
|
end
|
|
end
|
|
end
|
|
|
|
let(:admin) { FactoryBot.create(:admin) }
|
|
|
|
let(:post_attributes) do
|
|
{ secure: false }
|
|
end
|
|
|
|
let(:non_admin_post_attributes) do
|
|
post_attributes[:user] = FactoryBot.create(:user)
|
|
post_attributes
|
|
end
|
|
|
|
let(:admin_post_attributes) do
|
|
post_attributes[:user] = admin
|
|
post_attributes
|
|
end
|
|
|
|
context "with an admin posting" do
|
|
subject { FactoryBot.create(:post, admin_post_attributes) }
|
|
its(:secure) { should eq false }
|
|
end
|
|
|
|
context "with a non-admin posting" do
|
|
subject { FactoryBot.create(:post, non_admin_post_attributes) }
|
|
its(:secure) { should be_nil }
|
|
end
|
|
|
|
context "with no user posting" do
|
|
subject { FactoryBot.create(:post, post_attributes) }
|
|
its(:secure) { should be_nil }
|
|
end
|
|
end
|