mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
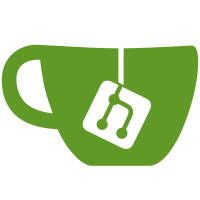
This commit deprecates several undocumented top-level FactoryBot methods: * callbacks * configuration * constructor * initialize_with * register_sequence * resent_configuration * skip_create * to_create factory_bot users are not meant to access these methods directly. Instead they are available for internal use through the new Internal module introduced in #1262. --- While addressing the new deprecation warnings, I tried to make the delegation a bit more consistent, always delegating to `FactoryBot::Internal`. This involved requiring "factory_bot/internal" sooner. To avoid having to fight with the order of requires and potential circular dependencies, I moved the default strategy and callback registration out of constants (this is how they used to be before #1297), so it doesn't matter if those strategies are loaded before the `Internal` module. I also deleted a pair of tests that were using these constants - these strategies and callbacks are covered elsewhere in our test suite, and I don't think these tests were adding much value (they missed #1379, for example).
64 lines
1.6 KiB
Ruby
64 lines
1.6 KiB
Ruby
module FactoryBot
|
|
module Syntax
|
|
module Default
|
|
include Methods
|
|
|
|
def define(&block)
|
|
DSL.run(block)
|
|
end
|
|
|
|
def modify(&block)
|
|
ModifyDSL.run(block)
|
|
end
|
|
|
|
class DSL
|
|
def factory(name, options = {}, &block)
|
|
factory = Factory.new(name, options)
|
|
proxy = FactoryBot::DefinitionProxy.new(factory.definition)
|
|
proxy.instance_eval(&block) if block_given?
|
|
|
|
Internal.register_factory(factory)
|
|
|
|
proxy.child_factories.each do |(child_name, child_options, child_block)|
|
|
parent_factory = child_options.delete(:parent) || name
|
|
factory(child_name, child_options.merge(parent: parent_factory), &child_block)
|
|
end
|
|
end
|
|
|
|
def sequence(name, *args, &block)
|
|
Internal.register_sequence(Sequence.new(name, *args, &block))
|
|
end
|
|
|
|
def trait(name, &block)
|
|
Internal.register_trait(Trait.new(name, &block))
|
|
end
|
|
|
|
def self.run(block)
|
|
new.instance_eval(&block)
|
|
end
|
|
|
|
delegate :after,
|
|
:before,
|
|
:callback,
|
|
:initialize_with,
|
|
:skip_create,
|
|
:to_create,
|
|
to: FactoryBot::Internal
|
|
end
|
|
|
|
class ModifyDSL
|
|
def factory(name, _options = {}, &block)
|
|
factory = Internal.factory_by_name(name)
|
|
proxy = FactoryBot::DefinitionProxy.new(factory.definition.overridable)
|
|
proxy.instance_eval(&block)
|
|
end
|
|
|
|
def self.run(block)
|
|
new.instance_eval(&block)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
extend Syntax::Default
|
|
end
|