mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
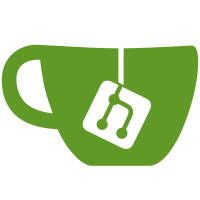
This introduces a couple of things: firstly, there's now a MockFactory that allows for introspection instead of spying all over the place. This should make tests a lot less brittle. Secondly, a couple of handy matchers have been made available to perform assertions against the mock.
42 lines
695 B
Ruby
42 lines
695 B
Ruby
class MockFactory
|
|
attr_reader :declarations, :traits
|
|
|
|
def initialize
|
|
@declarations = []
|
|
@traits = []
|
|
@callbacks = Hash.new([])
|
|
@to_create = nil
|
|
end
|
|
|
|
def to_create(&block)
|
|
if block_given?
|
|
@to_create = block
|
|
else
|
|
@to_create
|
|
end
|
|
end
|
|
|
|
def declare_attribute(declaration)
|
|
@declarations << declaration
|
|
end
|
|
|
|
def build_callbacks
|
|
@callbacks[:after_build]
|
|
end
|
|
|
|
def create_callbacks
|
|
@callbacks[:after_create]
|
|
end
|
|
|
|
def stub_callbacks
|
|
@callbacks[:after_stub]
|
|
end
|
|
|
|
def add_callback(callback_type, &callback)
|
|
@callbacks[callback_type] << callback
|
|
end
|
|
|
|
def define_trait(trait)
|
|
@traits << trait
|
|
end
|
|
end
|