mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
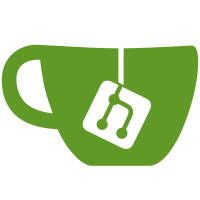
Before this PR, `use_parent_strategy` was set on the configuration instance. Since `FactoryBot.reload` wipes out the configuration, it also ends up resetting `use_parent_strategy` back to `nil`. This can cause problems when using factory_bot_rails with Spring, since it calls `FactoryBot.reload` each time Spring forks. If `use_parent_strategy` is set in a file that Spring preloads, like in an initializer, then the value will get wiped out. With this PR, we set `use_parent_strategy` directly on FactoryBot, rather than on the configuration instance, and so reloading no longer has any effect.
97 lines
2 KiB
Ruby
97 lines
2 KiB
Ruby
describe "a built instance" do
|
|
include FactoryBot::Syntax::Methods
|
|
|
|
before do
|
|
define_model("User")
|
|
|
|
define_model("Post", user_id: :integer) do
|
|
belongs_to :user
|
|
end
|
|
|
|
FactoryBot.define do
|
|
factory :user
|
|
|
|
factory :post do
|
|
user
|
|
end
|
|
end
|
|
end
|
|
|
|
subject { build(:post) }
|
|
|
|
it { should be_new_record }
|
|
|
|
context "when the :use_parent_strategy config option is set to false" do
|
|
before { FactoryBot.use_parent_strategy = false }
|
|
|
|
it "assigns and saves associations" do
|
|
expect(subject.user).to be_kind_of(User)
|
|
expect(subject.user).not_to be_new_record
|
|
end
|
|
end
|
|
|
|
context "when the :use_parent_strategy config option is set to true" do
|
|
before { FactoryBot.use_parent_strategy = true }
|
|
|
|
it "assigns but does not save associations" do
|
|
expect(subject.user).to be_kind_of(User)
|
|
expect(subject.user).to be_new_record
|
|
end
|
|
end
|
|
end
|
|
|
|
describe "a built instance with strategy: :create" do
|
|
include FactoryBot::Syntax::Methods
|
|
|
|
before do
|
|
define_model("User")
|
|
|
|
define_model("Post", user_id: :integer) do
|
|
belongs_to :user
|
|
end
|
|
|
|
FactoryBot.define do
|
|
factory :user
|
|
|
|
factory :post do
|
|
association(:user, strategy: :create)
|
|
end
|
|
end
|
|
end
|
|
|
|
subject { build(:post) }
|
|
|
|
it { should be_new_record }
|
|
|
|
it "assigns and saves associations" do
|
|
expect(subject.user).to be_kind_of(User)
|
|
expect(subject.user).not_to be_new_record
|
|
end
|
|
end
|
|
|
|
describe "calling `build` with a block" do
|
|
include FactoryBot::Syntax::Methods
|
|
|
|
before do
|
|
define_model("Company", name: :string)
|
|
|
|
FactoryBot.define do
|
|
factory :company
|
|
end
|
|
end
|
|
|
|
it "passes the built instance" do
|
|
build(:company, name: "thoughtbot") do |company|
|
|
expect(company.name).to eq("thoughtbot")
|
|
end
|
|
end
|
|
|
|
it "returns the built instance" do
|
|
expected = nil
|
|
result = build(:company) do |company|
|
|
expected = company
|
|
"hello!"
|
|
end
|
|
expect(result).to eq expected
|
|
end
|
|
end
|