mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
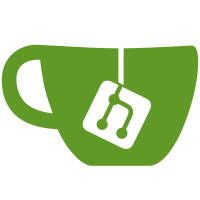
Closes https://github.com/thoughtbot/factory_bot/issues/1227 In this code we are passing an implicit declaration `user`, rather than the symbol `:user`: ```rb factory :post do author factory: user end ``` In https://github.com/thoughtbot/factory_bot/issues/1227 we improved the error message from: ```rb undefined method 'name' for :post:Symbol ``` to: ```rb Trait not registered: user ``` But this still doesn't make it totally obvious what the error was. Why are we trying to register a user trait? With this PR we update the error message to: ```rb Association 'author' received an invalid factory argument. Did you mean? 'factory: :user' ```
41 lines
1 KiB
Ruby
41 lines
1 KiB
Ruby
module FactoryBot
|
|
class Declaration
|
|
# @api private
|
|
class Association < Declaration
|
|
def initialize(name, *options)
|
|
super(name, false)
|
|
@options = options.dup
|
|
@overrides = options.extract_options!
|
|
@traits = options
|
|
end
|
|
|
|
def ==(other)
|
|
self.class == other.class &&
|
|
name == other.name &&
|
|
options == other.options
|
|
end
|
|
|
|
protected
|
|
|
|
attr_reader :options
|
|
|
|
private
|
|
|
|
def build
|
|
ensure_factory_is_not_a_declaration!
|
|
|
|
factory_name = @overrides[:factory] || name
|
|
[Attribute::Association.new(name, factory_name, [@traits, @overrides.except(:factory)].flatten)]
|
|
end
|
|
|
|
def ensure_factory_is_not_a_declaration!
|
|
if @overrides[:factory].is_a?(Declaration)
|
|
raise ArgumentError.new(<<~MSG)
|
|
Association '#{name}' received an invalid factory argument.
|
|
Did you mean? 'factory: :#{@overrides[:factory].name}'
|
|
MSG
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|