mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
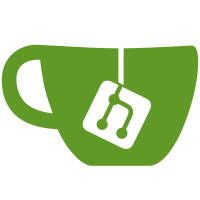
Before this commit, factory_bot registered default callbacks in a global
registry, and offered a `register_callback` method for registering any
custom callbacks (which would be fired within custom strategies).
The idea here seems to have been that we could check against this global
registry and then raise an error when referring to a callback that was
not registered.
However, nobody ever saw this error because we have always had the line:
`FactoryBot::Internal.register_callback(name)`, which registers
callbacks on the fly whenever they are referred to. (I noticed this when
a [change to default callbacks] accidentally duplicated `after_create`
instead of including `before_create`, and we didn't get any tests
failures.)
We have two options here:
1. Preserve the existing behavior by deleting all the callback
registration code. (That is what this commit does)
2. Change to the code try and capture what I assume was the original
intention. This would be a breaking change, so we would want to
introduce it in a major release. (See #1379)
I prefer preserving the existing behavior because we haven't seen any
issues around this, and making a breaking change for this doesn't seem
worthwhile.
[change to default callbacks]: f82e40c8c5 (diff-c6d30be672f880311a7df0820dc4fb21R12-R14)
91 lines
2.2 KiB
Ruby
91 lines
2.2 KiB
Ruby
module FactoryBot
|
|
# @api private
|
|
module Internal
|
|
class << self
|
|
delegate :after,
|
|
:before,
|
|
:callbacks,
|
|
:constructor,
|
|
:factories,
|
|
:initialize_with,
|
|
:inline_sequences,
|
|
:sequences,
|
|
:skip_create,
|
|
:strategies,
|
|
:to_create,
|
|
:traits,
|
|
to: :configuration
|
|
|
|
def configuration
|
|
@configuration ||= Configuration.new
|
|
end
|
|
|
|
def reset_configuration
|
|
@configuration = nil
|
|
end
|
|
|
|
def register_inline_sequence(sequence)
|
|
inline_sequences.push(sequence)
|
|
end
|
|
|
|
def rewind_inline_sequences
|
|
inline_sequences.each(&:rewind)
|
|
end
|
|
|
|
def register_trait(trait)
|
|
trait.names.each do |name|
|
|
traits.register(name, trait)
|
|
end
|
|
trait
|
|
end
|
|
|
|
def trait_by_name(name)
|
|
traits.find(name)
|
|
end
|
|
|
|
def register_sequence(sequence)
|
|
sequence.names.each do |name|
|
|
sequences.register(name, sequence)
|
|
end
|
|
sequence
|
|
end
|
|
|
|
def sequence_by_name(name)
|
|
sequences.find(name)
|
|
end
|
|
|
|
def rewind_sequences
|
|
sequences.each(&:rewind)
|
|
rewind_inline_sequences
|
|
end
|
|
|
|
def register_factory(factory)
|
|
factory.names.each do |name|
|
|
factories.register(name, factory)
|
|
end
|
|
factory
|
|
end
|
|
|
|
def factory_by_name(name)
|
|
factories.find(name)
|
|
end
|
|
|
|
def register_strategy(strategy_name, strategy_class)
|
|
strategies.register(strategy_name, strategy_class)
|
|
StrategySyntaxMethodRegistrar.new(strategy_name).define_strategy_methods
|
|
end
|
|
|
|
def strategy_by_name(name)
|
|
strategies.find(name)
|
|
end
|
|
|
|
def register_default_strategies
|
|
register_strategy(:build, FactoryBot::Strategy::Build)
|
|
register_strategy(:create, FactoryBot::Strategy::Create)
|
|
register_strategy(:attributes_for, FactoryBot::Strategy::AttributesFor)
|
|
register_strategy(:build_stubbed, FactoryBot::Strategy::Stub)
|
|
register_strategy(:null, FactoryBot::Strategy::Null)
|
|
end
|
|
end
|
|
end
|
|
end
|