mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
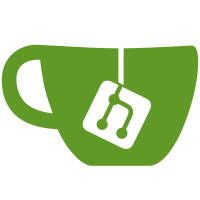
* Alphabetize gem listing in various Gemfiles [Rubocop Bundler/OrderedGems] * Fix alignment of if/else/end statement [Rubocop Layout/ElseAlignment] * Method definitions should have a empty line between them [Rubocop Layout/EmptyLineBetweenDefs] * Modules, Classes, and blocks should have an empty line around them [Rubocop] Cops: Layout/EmptyLinesAroundBlockBody Layout/EmptyLinesAroundModuleBody Layout/EmptyLinesAroundClassBody Layout/EmptyLinesAroundAccessModifier * Keep a blank line before and after access modifiers [Rubocop Layout/EmptyLinesAroundAccessModifier] * Remove misc extra whitespace [Rubocop Layout/ExtraSpacing] * Indent the first line of the right-hand-side of a multi-line assignment [Rubocop Layout/IndentAssignment] * Remove extraneous whitespace [Rubocop] Cops: Layout/IndentationWidth Layout/LeadingCommentSpace Layout/SpaceAroundEqualsInParameterDefault Layout/SpaceInsideArrayLiteralBrackets Layout/SpaceInsideBlockBraces Layout/SpaceInsideParens Layout/TrailingBlankLines * Revert rubocop changes to gemfiles; exclude files from rubocop checks The files in gemfiles/ are generated by Appraisal, so we shouldn't edit them. Instead, let's tell RuboCop to exclude this directory.
70 lines
1.2 KiB
Ruby
70 lines
1.2 KiB
Ruby
module FactoryBot
|
|
# Sequences are defined using sequence within a FactoryBot.define block.
|
|
# Sequence values are generated using next.
|
|
# @api private
|
|
class Sequence
|
|
attr_reader :name
|
|
|
|
def initialize(name, *args, &proc)
|
|
@name = name
|
|
@proc = proc
|
|
|
|
options = args.extract_options!
|
|
@value = args.first || 1
|
|
@aliases = options.fetch(:aliases) { [] }
|
|
|
|
if !@value.respond_to?(:peek)
|
|
@value = EnumeratorAdapter.new(@value)
|
|
end
|
|
end
|
|
|
|
def next(scope = nil)
|
|
if @proc && scope
|
|
scope.instance_exec(value, &@proc)
|
|
elsif @proc
|
|
@proc.call(value)
|
|
else
|
|
value
|
|
end
|
|
ensure
|
|
increment_value
|
|
end
|
|
|
|
def names
|
|
[@name] + @aliases
|
|
end
|
|
|
|
def rewind
|
|
@value.rewind
|
|
end
|
|
|
|
private
|
|
|
|
def value
|
|
@value.peek
|
|
end
|
|
|
|
def increment_value
|
|
@value.next
|
|
end
|
|
|
|
class EnumeratorAdapter
|
|
def initialize(value)
|
|
@first_value = value
|
|
@value = value
|
|
end
|
|
|
|
def peek
|
|
@value
|
|
end
|
|
|
|
def next
|
|
@value = @value.next
|
|
end
|
|
|
|
def rewind
|
|
@value = @first_value
|
|
end
|
|
end
|
|
end
|
|
end
|