mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
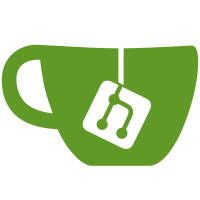
Most of this was fixed by adding the `attribute-defined-statically-cop` branch of `thoughtbot/rubocop-rspec` to the Gemfile and running: ```sh rubocop \ --require rubocop-rspec \ --only FactoryBot/AttributeDefinedStatically \ --auto-correct ``` I had to update the cucumber tests manually, and I realized our changes don't handle `ignore` blocks or blocks with arity 1 that use the yielded DefinitionProxy. I will update https://github.com/rubocop-hq/rubocop-rspec/pull/666to handle these cases.
49 lines
1.5 KiB
Ruby
49 lines
1.5 KiB
Ruby
describe "a generated attributes hash where order matters" do
|
|
include FactoryBot::Syntax::Methods
|
|
|
|
before do
|
|
define_model('ParentModel', static: :integer,
|
|
evaluates_first: :integer,
|
|
evaluates_second: :integer,
|
|
evaluates_third: :integer)
|
|
|
|
FactoryBot.define do
|
|
factory :parent_model do
|
|
evaluates_first { static }
|
|
evaluates_second { evaluates_first }
|
|
evaluates_third { evaluates_second }
|
|
|
|
factory :child_model do
|
|
static { 1 }
|
|
end
|
|
end
|
|
|
|
factory :without_parent, class: ParentModel do
|
|
evaluates_first { static }
|
|
evaluates_second { evaluates_first }
|
|
evaluates_third { evaluates_second }
|
|
static { 1 }
|
|
end
|
|
end
|
|
end
|
|
|
|
context "factory with a parent" do
|
|
subject { FactoryBot.build(:child_model) }
|
|
|
|
it "assigns attributes in the order they're defined with preference to static attributes" do
|
|
expect(subject[:evaluates_first]).to eq 1
|
|
expect(subject[:evaluates_second]).to eq 1
|
|
expect(subject[:evaluates_third]).to eq 1
|
|
end
|
|
end
|
|
|
|
context "factory without a parent" do
|
|
subject { FactoryBot.build(:without_parent) }
|
|
|
|
it "assigns attributes in the order they're defined with preference to static attributes without a parent class" do
|
|
expect(subject[:evaluates_first]).to eq 1
|
|
expect(subject[:evaluates_second]).to eq 1
|
|
expect(subject[:evaluates_third]).to eq 1
|
|
end
|
|
end
|
|
end
|