mirror of
https://github.com/thoughtbot/factory_bot.git
synced 2022-11-09 11:43:51 -05:00
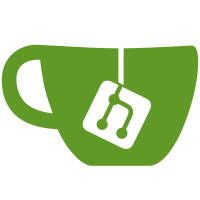
Closes #1391 Along the same lines as #1286, this commit raises a more helpful error if somebody passes an implicit declaration as an association argument. Before this commit, an association with an implicit trait passed as an override attribute: ```rb person factory: :user, invalid_attribute: implicit_trait ``` Would raise an error `KeyError: Trait not registered: "implicit_trait"`. This is potentially confusing, since the author probably didn't intend to define an implicit trait. After this commit, this will raise a more helpful error: ``` ArgumentError: Association 'person' received an invalid attribute override. Did you mean? 'invalid_attribute}: :implicit_trait}' ```
58 lines
1.4 KiB
Ruby
58 lines
1.4 KiB
Ruby
module FactoryBot
|
|
class Declaration
|
|
# @api private
|
|
class Association < Declaration
|
|
def initialize(name, *options)
|
|
super(name, false)
|
|
@options = options.dup
|
|
@overrides = options.extract_options!
|
|
@factory_name = @overrides.delete(:factory) || name
|
|
@traits = options
|
|
end
|
|
|
|
def ==(other)
|
|
self.class == other.class &&
|
|
name == other.name &&
|
|
options == other.options
|
|
end
|
|
|
|
protected
|
|
|
|
attr_reader :options
|
|
|
|
private
|
|
|
|
attr_reader :factory_name, :overrides, :traits
|
|
|
|
def build
|
|
raise_if_arguments_are_declarations!
|
|
|
|
[
|
|
Attribute::Association.new(
|
|
name,
|
|
factory_name,
|
|
[traits, overrides].flatten
|
|
)
|
|
]
|
|
end
|
|
|
|
def raise_if_arguments_are_declarations!
|
|
if factory_name.is_a?(Declaration)
|
|
raise ArgumentError.new(<<~MSG)
|
|
Association '#{name}' received an invalid factory argument.
|
|
Did you mean? 'factory: :#{factory_name.name}'
|
|
MSG
|
|
end
|
|
|
|
overrides.each do |attribute, value|
|
|
if value.is_a?(Declaration)
|
|
raise ArgumentError.new(<<~MSG)
|
|
Association '#{name}' received an invalid attribute override.
|
|
Did you mean? '#{attribute}: :#{value.name}'
|
|
MSG
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|