mirror of
https://github.com/thoughtbot/factory_bot_rails.git
synced 2022-11-09 11:49:18 -05:00
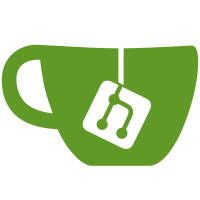
Fixes #165 and closes #166 Currently the only way to customize the factory definition file paths is to do it in an initializer right after the "factory_bot.set_factory_paths" initializer. With this commit, we can customize factory definition file paths by setting `config.factory_bot.definition_file_paths` in config/application.rb or the appropriate environment file. Once we update the documentation to include this new configuration, we should be able to close #149 and #180 as well, since using this configuration can replace the initializer solution for sharing factories in an engine (the initializer solution will still work, but with the new configuration you don't need to know anything about the fbr railtie). This will also allow us to close #192, since we can use this configuration with an empty array to disable automatic loading of factories in development.
153 lines
4.6 KiB
Gherkin
153 lines
4.6 KiB
Gherkin
Feature: automatically load factory definitions
|
|
|
|
Background:
|
|
When I successfully run `bundle exec rails new testapp`
|
|
And I cd to "testapp"
|
|
And I add "factory_bot_rails" from this project as a dependency
|
|
And I add "test-unit" as a dependency
|
|
And I run `bundle install` with a clean environment
|
|
And I write to "db/migrate/1_create_users.rb" with:
|
|
"""
|
|
migration_class =
|
|
if ActiveRecord::Migration.respond_to?(:[])
|
|
ActiveRecord::Migration[4.2]
|
|
else
|
|
ActiveRecord::Migration
|
|
end
|
|
|
|
class CreateUsers < migration_class
|
|
def self.up
|
|
create_table :users do |t|
|
|
t.string :name
|
|
end
|
|
end
|
|
end
|
|
"""
|
|
When I run `bundle exec rake db:migrate` with a clean environment
|
|
And I write to "app/models/user.rb" with:
|
|
"""
|
|
class User < ActiveRecord::Base
|
|
end
|
|
"""
|
|
|
|
Scenario: generate a Rails application and use factory definitions
|
|
When I write to "test/factories.rb" with:
|
|
"""
|
|
FactoryBot.define do
|
|
factory :user do
|
|
name { "Frank" }
|
|
end
|
|
end
|
|
"""
|
|
When I write to "test/unit/user_test.rb" with:
|
|
"""
|
|
require 'test_helper'
|
|
|
|
class UserTest < ActiveSupport::TestCase
|
|
test "use factory" do
|
|
user = FactoryBot.create(:user)
|
|
assert_equal 'Frank', user.name
|
|
end
|
|
end
|
|
"""
|
|
When I run `bundle exec rake test` with a clean environment
|
|
Then the output should contain "1 assertions, 0 failures, 0 errors"
|
|
|
|
Scenario: use custom definition file paths
|
|
When I configure the factories as:
|
|
"""
|
|
config.factory_bot.definition_file_paths = ["custom_factories_path"]
|
|
"""
|
|
When I write to "custom_factories_path.rb" with:
|
|
"""
|
|
FactoryBot.define do
|
|
factory :user do
|
|
name { "Frank" }
|
|
end
|
|
end
|
|
"""
|
|
When I write to "test/unit/user_test.rb" with:
|
|
"""
|
|
require 'test_helper'
|
|
|
|
class UserTest < ActiveSupport::TestCase
|
|
test "use factory" do
|
|
user = FactoryBot.create(:user)
|
|
assert_equal 'Frank', user.name
|
|
end
|
|
end
|
|
"""
|
|
When I run `bundle exec rake test` with a clean environment
|
|
Then the output should contain "1 assertions, 0 failures, 0 errors"
|
|
|
|
Scenario: use 3rd-party factories with configured definition file paths
|
|
When I append to "config/application.rb" with:
|
|
"""
|
|
require File.expand_path('../../lib/some_railtie/railties.rb', __FILE__)
|
|
"""
|
|
When I write to "lib/some_railtie/railties.rb" with:
|
|
"""
|
|
module SomeRailtie
|
|
class Railtie < ::Rails::Engine
|
|
config.factory_bot.definition_file_paths << File.expand_path('../factories', __FILE__)
|
|
end
|
|
end
|
|
"""
|
|
When I write to "lib/some_railtie/factories.rb" with:
|
|
"""
|
|
FactoryBot.define do
|
|
factory :factory_from_some_railtie, class: 'User' do
|
|
name { 'Artem' }
|
|
end
|
|
end
|
|
"""
|
|
When I write to "test/unit/user_test.rb" with:
|
|
"""
|
|
require 'test_helper'
|
|
|
|
class UserTest < ActiveSupport::TestCase
|
|
test "use factory of some_railtie" do
|
|
user = FactoryBot.create(:factory_from_some_railtie)
|
|
assert_equal 'Artem', user.name
|
|
end
|
|
end
|
|
"""
|
|
When I run `bundle exec rake test` with a clean environment
|
|
Then the output should contain "1 assertions, 0 failures, 0 errors"
|
|
|
|
Scenario: use 3rd-party factories with an initializer
|
|
When I append to "config/application.rb" with:
|
|
"""
|
|
require File.expand_path('../../lib/some_railtie/railties.rb', __FILE__)
|
|
"""
|
|
When I write to "lib/some_railtie/railties.rb" with:
|
|
"""
|
|
module SomeRailtie
|
|
class Railtie < ::Rails::Engine
|
|
initializer "some_railtie.factories", :after => "factory_bot.set_factory_paths" do
|
|
FactoryBot.definition_file_paths << File.expand_path('../factories', __FILE__)
|
|
end
|
|
end
|
|
end
|
|
"""
|
|
When I write to "lib/some_railtie/factories.rb" with:
|
|
"""
|
|
FactoryBot.define do
|
|
factory :factory_from_some_railtie, class: 'User' do
|
|
name { 'Artem' }
|
|
end
|
|
end
|
|
"""
|
|
When I write to "test/unit/user_test.rb" with:
|
|
"""
|
|
require 'test_helper'
|
|
|
|
class UserTest < ActiveSupport::TestCase
|
|
test "use factory of some_railtie" do
|
|
user = FactoryBot.create(:factory_from_some_railtie)
|
|
assert_equal 'Artem', user.name
|
|
end
|
|
end
|
|
"""
|
|
When I run `bundle exec rake test` with a clean environment
|
|
Then the output should contain "1 assertions, 0 failures, 0 errors"
|