mirror of
https://github.com/thoughtbot/shoulda-matchers.git
synced 2022-11-09 12:01:38 -05:00
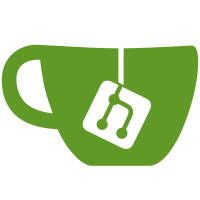
`allow_value` matcher is, of course, concerned with setting values on a particular attribute on a particular record, and then checking that the record is valid after doing so. That comes with a caveat: if the attribute is overridden in such a way so that the same value going into the attribute isn't the same value coming out of it, then `allow_value` will balk -- it'll say, "I can't do that because that changes how I work." That's all well and good, but what the attribute intentionally changes incoming values? ActiveRecord's typecasting behavior, for instance, would trigger such an exception. What if the developer needs a way to get around this? This is where `ignoring_interference_by_writer` comes into play. You can tack it on to the end of the matcher, and you're free to go on your way. So, prior to this commit you could already apply it to `allow_value`, but now in this commit it also works on any other matcher. But, one little thing: sometimes using this qualifier isn't going to work. Perhaps you or something else actually *is* overriding the attribute to change incoming values in a specific way, and perhaps the value that comes out makes the record fail validation, and there's nothing you can do about it. So in this case, even if you're using `ignoring_interference_by_writer`, we want to inform you about what the attribute is doing -- what the input and output was. And so we do.
55 lines
1.5 KiB
Ruby
55 lines
1.5 KiB
Ruby
require 'acceptance_spec_helper'
|
|
|
|
describe 'shoulda-matchers integrates with multiple libraries' do
|
|
before do
|
|
create_rails_application
|
|
|
|
write_file 'db/migrate/1_create_users.rb', <<-FILE
|
|
class CreateUsers < ActiveRecord::Migration
|
|
def self.up
|
|
create_table :users do |t|
|
|
t.string :name
|
|
end
|
|
end
|
|
end
|
|
FILE
|
|
|
|
run_rake_tasks!(*%w(db:drop db:create db:migrate))
|
|
|
|
write_file 'app/models/user.rb', <<-FILE
|
|
class User < ActiveRecord::Base
|
|
validates_presence_of :name
|
|
validates_uniqueness_of :name
|
|
end
|
|
FILE
|
|
|
|
add_rspec_file 'spec/models/user_spec.rb', <<-FILE
|
|
describe User do
|
|
subject { User.new(name: "John Smith") }
|
|
it { should validate_presence_of(:name) }
|
|
it { should validate_uniqueness_of(:name) }
|
|
end
|
|
FILE
|
|
|
|
updating_bundle do
|
|
add_rspec_rails_to_project!
|
|
add_shoulda_matchers_to_project(
|
|
test_frameworks: [:rspec],
|
|
libraries: [:active_record, :active_model]
|
|
)
|
|
end
|
|
end
|
|
|
|
context 'when using both active_record and active_model libraries' do
|
|
it 'allows the use of matchers from both libraries' do
|
|
result = run_rspec_suite
|
|
expect(result).to have_output('2 examples, 0 failures')
|
|
expect(result).to have_output(
|
|
'should validate that :name cannot be empty/falsy'
|
|
)
|
|
expect(result).to have_output(
|
|
'should validate that :name is case-sensitively unique'
|
|
)
|
|
end
|
|
end
|
|
end
|