mirror of
https://github.com/thoughtbot/shoulda-matchers.git
synced 2022-11-09 12:01:38 -05:00
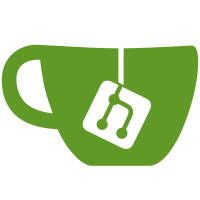
This is part of a collection of commits that aim to improve failure messages across the board, in order to make matchers easier to debug when something goes wrong. * Have the failure message describe more clearly what the `allow_value` matcher was trying to do when it failed. * Make the description of the matcher more readable. * For each value that `allow_value` sets, use a different Validator instance. The matcher still changes state as it runs, but a future commit will refactor this further. * Merge StrictValidator back into Validator, and remove it. The way that StrictValidator worked (as a module that was mixed into an instance of Validator at runtime) was confusing, and there's really no need to split out the logic anymore. * Fix or fill in tests involving failure messages and descriptions.
67 lines
1.4 KiB
Ruby
67 lines
1.4 KiB
Ruby
require 'forwardable'
|
|
|
|
module Shoulda
|
|
module Matchers
|
|
module ActiveModel
|
|
# @private
|
|
class DisallowValueMatcher
|
|
extend Forwardable
|
|
|
|
def_delegators :allow_matcher,
|
|
:_after_setting_value,
|
|
:attribute_to_set,
|
|
:description,
|
|
:model,
|
|
:last_value_set,
|
|
:simple_description,
|
|
:failure_message_preface,
|
|
:failure_message_preface=
|
|
|
|
def initialize(value)
|
|
@allow_matcher = AllowValueMatcher.new(value)
|
|
end
|
|
|
|
def matches?(subject)
|
|
!allow_matcher.matches?(subject)
|
|
end
|
|
|
|
def for(attribute)
|
|
allow_matcher.for(attribute)
|
|
self
|
|
end
|
|
|
|
def on(context)
|
|
allow_matcher.on(context)
|
|
self
|
|
end
|
|
|
|
def with_message(message, options={})
|
|
allow_matcher.with_message(message, options)
|
|
self
|
|
end
|
|
|
|
def strict(strict = true)
|
|
allow_matcher.strict(strict)
|
|
self
|
|
end
|
|
|
|
def ignoring_interference_by_writer
|
|
allow_matcher.ignoring_interference_by_writer
|
|
self
|
|
end
|
|
|
|
def failure_message
|
|
allow_matcher.failure_message_when_negated
|
|
end
|
|
|
|
def failure_message_when_negated
|
|
allow_matcher.failure_message
|
|
end
|
|
|
|
protected
|
|
|
|
attr_reader :allow_matcher
|
|
end
|
|
end
|
|
end
|
|
end
|