mirror of
https://github.com/thoughtbot/shoulda-matchers.git
synced 2022-11-09 12:01:38 -05:00
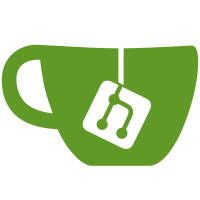
In Rails 4.2, ActiveRecord was changed such that if you attempt to set an attribute to a value and that value is outside the range of the column, then it will raise a RangeError. For instance, an integer column with a limit of 2 (i.e. a smallint) only accepts values between -32768 and +32767. This means that if you try to do any of these three things, a RangeError could be raised: * Use validate_numericality_of along with any of the comparison submatchers and a value that sits on either side of the boundary. * Use allow_value with a value that sits outside the range. * Use validates_inclusion_of against an integer column. (Here we attempt to set that column to a non-integer value to verify that the attribute does not allow said value. That value is really a string version of a large number, so if the column does not take large numbers then the matcher could blow up.) Ancillary changes in this commit: * Remove ValidationMessageFinder and ExceptionMessageFinder in favor of Validator, StrictValidator, and ValidatorWithCapturedRangeError. * The allow_value matcher now uses an instance of Validator under the hood. StrictValidator and/or ValidatorWithCapturedRangeError may be mixed into the Validator object as needed.
28 lines
709 B
Ruby
28 lines
709 B
Ruby
module UnitTests
|
|
module ActiveModelVersions
|
|
def self.configure_example_group(example_group)
|
|
example_group.include(self)
|
|
example_group.extend(self)
|
|
end
|
|
|
|
def active_model_version
|
|
Tests::Version.new(::ActiveModel::VERSION::STRING)
|
|
end
|
|
|
|
def active_model_3_1?
|
|
(::ActiveModel::VERSION::MAJOR == 3 && ::ActiveModel::VERSION::MINOR >= 1) || active_model_4_0?
|
|
end
|
|
|
|
def active_model_3_2?
|
|
(::ActiveModel::VERSION::MAJOR == 3 && ::ActiveModel::VERSION::MINOR >= 2) || active_model_4_0?
|
|
end
|
|
|
|
def active_model_4_0?
|
|
::ActiveModel::VERSION::MAJOR == 4
|
|
end
|
|
|
|
def active_model_supports_strict?
|
|
active_model_version >= 3.2
|
|
end
|
|
end
|
|
end
|