mirror of
https://github.com/thoughtbot/shoulda-matchers.git
synced 2022-11-09 12:01:38 -05:00
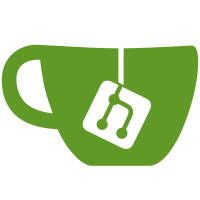
* Use `permit` directly instead of manually building the matcher * Some tests were being tested by calling #matches? directly when they shouldn't have been, and some tests were duplicated * Rename helper methods that define controllers to express intent better * Don't make a global class (SimulatedError), keep the class local to the example group * Use lambdas for that use `raise_error`
76 lines
1.9 KiB
Ruby
76 lines
1.9 KiB
Ruby
module UnitTests
|
|
module ClassBuilder
|
|
def self.parse_constant_name(name)
|
|
namespace = Shoulda::Matchers::Util.deconstantize(name)
|
|
qualified_namespace = (namespace.presence || 'Object').constantize
|
|
name_without_namespace = name.to_s.demodulize
|
|
[qualified_namespace, name_without_namespace]
|
|
end
|
|
|
|
def self.configure_example_group(example_group)
|
|
example_group.include(self)
|
|
|
|
example_group.after do
|
|
teardown_defined_constants
|
|
end
|
|
end
|
|
|
|
def define_module(module_name, &block)
|
|
module_name = module_name.to_s.camelize
|
|
|
|
namespace, name_without_namespace =
|
|
ClassBuilder.parse_constant_name(module_name)
|
|
|
|
if namespace.const_defined?(name_without_namespace, false)
|
|
namespace.__send__(:remove_const, name_without_namespace)
|
|
end
|
|
|
|
eval <<-RUBY
|
|
module #{namespace}::#{name_without_namespace}
|
|
end
|
|
RUBY
|
|
|
|
namespace.const_get(name_without_namespace).tap do |constant|
|
|
constant.unloadable
|
|
|
|
if block
|
|
constant.module_eval(&block)
|
|
end
|
|
end
|
|
end
|
|
|
|
def define_class(class_name, parent_class = Object, &block)
|
|
class_name = class_name.to_s.camelize
|
|
|
|
namespace, name_without_namespace =
|
|
ClassBuilder.parse_constant_name(class_name)
|
|
|
|
if namespace.const_defined?(name_without_namespace, false)
|
|
namespace.__send__(:remove_const, name_without_namespace)
|
|
end
|
|
|
|
eval <<-RUBY
|
|
class #{namespace}::#{name_without_namespace} < #{parent_class}
|
|
end
|
|
RUBY
|
|
|
|
namespace.const_get(name_without_namespace).tap do |constant|
|
|
constant.unloadable
|
|
|
|
if block
|
|
if block.arity == 0
|
|
constant.class_eval(&block)
|
|
else
|
|
block.call(constant)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def teardown_defined_constants
|
|
ActiveSupport::Dependencies.clear
|
|
end
|
|
end
|
|
end
|