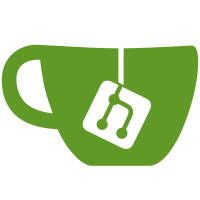
Prior to GitLab 9.0, attachments were not tracked the `uploads` table, so it was possible that the appearance logos were just stored in the database as a string and mounted via CarrierWave. https://gitlab.com/gitlab-org/gitlab-ce/issues/29240 implemented in GitLab 10.3 was supposed to cover populating the `uploads` table for all attachments, including all the logos from appearances. However, it's possible that didn't work for logos or the `uploads` entry was orphaned. GitLab instances that had a customized logo with no associated `uploads` entry would see Error 500s. The only way to fix this is to delete the `logo` column from the `appearances` table and re-upload the attachment. This change makes things more robust by falling back to the original behavior if the upload is not available. This is a CE backport of https://gitlab.com/gitlab-org/gitlab-ee/merge_requests/9277. Closes https://gitlab.com/gitlab-org/gitlab-ee/issues/9357
63 lines
1.7 KiB
Ruby
63 lines
1.7 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
class Appearance < ActiveRecord::Base
|
|
include CacheableAttributes
|
|
include CacheMarkdownField
|
|
include ObjectStorage::BackgroundMove
|
|
include WithUploads
|
|
|
|
cache_markdown_field :description
|
|
cache_markdown_field :new_project_guidelines
|
|
|
|
validates :logo, file_size: { maximum: 1.megabyte }
|
|
validates :header_logo, file_size: { maximum: 1.megabyte }
|
|
|
|
validate :single_appearance_row, on: :create
|
|
|
|
mount_uploader :logo, AttachmentUploader
|
|
mount_uploader :header_logo, AttachmentUploader
|
|
mount_uploader :favicon, FaviconUploader
|
|
|
|
# Overrides CacheableAttributes.current_without_cache
|
|
def self.current_without_cache
|
|
first
|
|
end
|
|
|
|
def single_appearance_row
|
|
if self.class.any?
|
|
errors.add(:single_appearance_row, 'Only 1 appearances row can exist')
|
|
end
|
|
end
|
|
|
|
def logo_path
|
|
logo_system_path(logo, 'logo')
|
|
end
|
|
|
|
def header_logo_path
|
|
logo_system_path(header_logo, 'header_logo')
|
|
end
|
|
|
|
def favicon_path
|
|
logo_system_path(favicon, 'favicon')
|
|
end
|
|
|
|
private
|
|
|
|
def logo_system_path(logo, mount_type)
|
|
# Legacy attachments may not have have an associated Upload record,
|
|
# so fallback to the AttachmentUploader#url if this is the
|
|
# case. AttachmentUploader#path doesn't work because for a local
|
|
# file, this is an absolute path to the file.
|
|
return logo&.url unless logo&.upload
|
|
|
|
# If we're using a CDN, we need to use the full URL
|
|
asset_host = ActionController::Base.asset_host
|
|
local_path = Gitlab::Routing.url_helpers.appearance_upload_path(
|
|
filename: logo.filename,
|
|
id: logo.upload.model_id,
|
|
model: 'appearance',
|
|
mounted_as: mount_type)
|
|
|
|
Gitlab::Utils.append_path(asset_host, local_path)
|
|
end
|
|
end
|