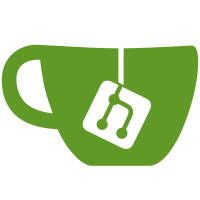
After installing a new gem, batch-loader, a construct can be used to queue data to be fetched in bulk. The gem was also introduced in both gitlab-org/gitlab-ce!14680 and gitlab-org/gitlab-ce!14846, but those mrs are not merged yet. For the generation of diffs, both the old blob and the new blob need to be loaded. This for every file in the diff, too. Now we collect all these so we do 1 fetch. Three `.allow_n_plus_1_calls` have been removed, which I expect to be valid, but this needs to be confirmed by a full CI run. Possibly closes: - https://gitlab.com/gitlab-org/gitlab-ce/issues/37445 - https://gitlab.com/gitlab-org/gitlab-ce/issues/37599 - https://gitlab.com/gitlab-org/gitlab-ce/issues/37431
144 lines
4.2 KiB
Ruby
144 lines
4.2 KiB
Ruby
require 'spec_helper'
|
|
|
|
describe DiffViewer::Base do
|
|
include FakeBlobHelpers
|
|
|
|
let(:project) { create(:project, :repository) }
|
|
let(:commit) { project.commit('570e7b2abdd848b95f2f578043fc23bd6f6fd24d') }
|
|
let(:diff_file) { commit.diffs.diff_file_with_new_path('files/ruby/popen.rb') }
|
|
|
|
let(:viewer_class) do
|
|
Class.new(described_class) do
|
|
include DiffViewer::ServerSide
|
|
|
|
self.extensions = %w(jpg)
|
|
self.binary = true
|
|
self.collapse_limit = 1.megabyte
|
|
self.size_limit = 5.megabytes
|
|
end
|
|
end
|
|
|
|
let(:viewer) { viewer_class.new(diff_file) }
|
|
|
|
describe '.can_render?' do
|
|
context 'when the extension is supported' do
|
|
let(:commit) { project.commit('2f63565e7aac07bcdadb654e253078b727143ec4') }
|
|
let(:diff_file) { commit.diffs.diff_file_with_new_path('files/images/6049019_460s.jpg') }
|
|
|
|
context 'when the binaryness matches' do
|
|
it 'returns true' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'when the binaryness does not match' do
|
|
let(:commit) { project.commit_by(oid: 'ae73cb07c9eeaf35924a10f713b364d32b2dd34f') }
|
|
let(:diff_file) { commit.diffs.diff_file_with_new_path('Gemfile.zip') }
|
|
|
|
it 'returns false' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'when the file type is supported' do
|
|
let(:commit) { project.commit('1a0b36b3cdad1d2ee32457c102a8c0b7056fa863') }
|
|
let(:diff_file) { commit.diffs.diff_file_with_new_path('LICENSE') }
|
|
|
|
before do
|
|
viewer_class.file_types = %i(license)
|
|
viewer_class.binary = false
|
|
end
|
|
|
|
context 'when the binaryness matches' do
|
|
it 'returns true' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'when the binaryness does not match' do
|
|
before do
|
|
allow_any_instance_of(Blob).to receive(:binary?).and_return(true)
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'when the extension and file type are not supported' do
|
|
it 'returns false' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_falsey
|
|
end
|
|
end
|
|
|
|
context 'when the file was renamed and only the old blob is supported' do
|
|
let(:commit) { project.commit_by(oid: '2f63565e7aac07bcdadb654e253078b727143ec4') }
|
|
let(:diff_file) { commit.diffs.diff_file_with_new_path('files/images/6049019_460s.jpg') }
|
|
|
|
before do
|
|
allow(diff_file).to receive(:renamed_file?).and_return(true)
|
|
viewer_class.extensions = %w(notjpg)
|
|
end
|
|
|
|
it 'returns false' do
|
|
expect(viewer_class.can_render?(diff_file)).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#collapsed?' do
|
|
context 'when the combined blob size is larger than the collapse limit' do
|
|
before do
|
|
allow(diff_file).to receive(:raw_size).and_return(1025.kilobytes)
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(viewer.collapsed?).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'when the combined blob size is smaller than the collapse limit' do
|
|
it 'returns false' do
|
|
expect(viewer.collapsed?).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#too_large?' do
|
|
context 'when the combined blob size is larger than the size limit' do
|
|
before do
|
|
allow(diff_file).to receive(:raw_size).and_return(6.megabytes)
|
|
end
|
|
|
|
it 'returns true' do
|
|
expect(viewer.too_large?).to be_truthy
|
|
end
|
|
end
|
|
|
|
context 'when the blob size is smaller than the size limit' do
|
|
it 'returns false' do
|
|
expect(viewer.too_large?).to be_falsey
|
|
end
|
|
end
|
|
end
|
|
|
|
describe '#render_error' do
|
|
context 'when the combined blob size is larger than the size limit' do
|
|
before do
|
|
allow(diff_file).to receive(:raw_size).and_return(6.megabytes)
|
|
end
|
|
|
|
it 'returns :too_large' do
|
|
expect(viewer.render_error).to eq(:too_large)
|
|
end
|
|
end
|
|
|
|
context 'when the combined blob size is smaller than the size limit' do
|
|
it 'returns nil' do
|
|
expect(viewer.render_error).to be_nil
|
|
end
|
|
end
|
|
end
|
|
end
|