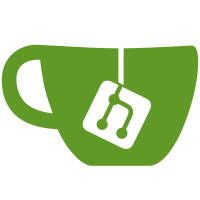
**Why?** It is significantly easier to manage the visibility of the modal in Vuex. The module contains the state and mutations to manage this. The component wraps GlModal and syncs the visibility with the module.
49 lines
1,009 B
JavaScript
49 lines
1,009 B
JavaScript
import mutations from '~/vuex_shared/modules/modal/mutations';
|
|
import * as types from '~/vuex_shared/modules/modal/mutation_types';
|
|
|
|
describe('Vuex ModalModule mutations', () => {
|
|
describe(types.SHOW, () => {
|
|
it('sets isVisible to true', () => {
|
|
const state = {
|
|
isVisible: false,
|
|
};
|
|
|
|
mutations[types.SHOW](state);
|
|
|
|
expect(state).toEqual({
|
|
isVisible: true,
|
|
});
|
|
});
|
|
});
|
|
|
|
describe(types.HIDE, () => {
|
|
it('sets isVisible to false', () => {
|
|
const state = {
|
|
isVisible: true,
|
|
};
|
|
|
|
mutations[types.HIDE](state);
|
|
|
|
expect(state).toEqual({
|
|
isVisible: false,
|
|
});
|
|
});
|
|
});
|
|
|
|
describe(types.OPEN, () => {
|
|
it('sets data and sets isVisible to true', () => {
|
|
const data = { id: 7 };
|
|
const state = {
|
|
isVisible: false,
|
|
data: null,
|
|
};
|
|
|
|
mutations[types.OPEN](state, data);
|
|
|
|
expect(state).toEqual({
|
|
isVisible: true,
|
|
data,
|
|
});
|
|
});
|
|
});
|
|
});
|