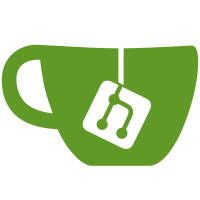
This refactors some of the logic used for protecting default branches, in particular Project#after_create_default_branch. The logic for this method is moved into a separate service class. Ideally we'd get rid of Project#after_create_default_branch entirely, but unfortunately Project#after_import depends on it. This means it has to stick around until we also refactor Project#after_import. For branch protection levels we introduce Gitlab::Access::BranchProtection, which provides a small wrapper around Integer based branch protection levels. Using this class removes the need for having to constantly refer to Gitlab::Access::PROTECTION_* constants.
67 lines
1.8 KiB
Ruby
67 lines
1.8 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module Projects
|
|
# Service class that can be used to execute actions necessary after creating a
|
|
# default branch.
|
|
class ProtectDefaultBranchService
|
|
attr_reader :project, :default_branch_protection
|
|
|
|
# @param [Project] project
|
|
def initialize(project)
|
|
@project = project
|
|
|
|
@default_branch_protection = Gitlab::Access::BranchProtection
|
|
.new(Gitlab::CurrentSettings.default_branch_protection)
|
|
end
|
|
|
|
def execute
|
|
protect_default_branch if default_branch
|
|
end
|
|
|
|
def protect_default_branch
|
|
# Ensure HEAD points to the default branch in case it is not master
|
|
project.change_head(default_branch)
|
|
|
|
create_protected_branch if protect_branch?
|
|
end
|
|
|
|
def create_protected_branch
|
|
params = {
|
|
name: default_branch,
|
|
push_access_levels_attributes: [{ access_level: push_access_level }],
|
|
merge_access_levels_attributes: [{ access_level: merge_access_level }]
|
|
}
|
|
|
|
# The creator of the project is always allowed to create protected
|
|
# branches, so we skip the authorization check in this service class.
|
|
ProtectedBranches::CreateService
|
|
.new(project, project.creator, params)
|
|
.execute(skip_authorization: true)
|
|
end
|
|
|
|
def protect_branch?
|
|
default_branch_protection.any? &&
|
|
!ProtectedBranch.protected?(project, default_branch)
|
|
end
|
|
|
|
def default_branch
|
|
project.default_branch
|
|
end
|
|
|
|
def push_access_level
|
|
if default_branch_protection.developer_can_push?
|
|
Gitlab::Access::DEVELOPER
|
|
else
|
|
Gitlab::Access::MAINTAINER
|
|
end
|
|
end
|
|
|
|
def merge_access_level
|
|
if default_branch_protection.developer_can_merge?
|
|
Gitlab::Access::DEVELOPER
|
|
else
|
|
Gitlab::Access::MAINTAINER
|
|
end
|
|
end
|
|
end
|
|
end
|