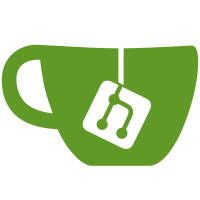
Dynamically adjust placedholder for uploads and fix Dropzone event handlers Override error handler to prevent error messages from being inserted underneath image preview Fix tests Use regexp instead of startsWith for better browser compatibility Remove duplicate code in _replace.html.haml and use one template Remove files upon error and retain alert messages until user adds a new file
314 lines
8.2 KiB
Ruby
314 lines
8.2 KiB
Ruby
# coding: utf-8
|
|
class Spinach::Features::ProjectSourceBrowseFiles < Spinach::FeatureSteps
|
|
include SharedAuthentication
|
|
include SharedProject
|
|
include SharedPaths
|
|
include RepoHelpers
|
|
|
|
step 'I should see files from repository' do
|
|
expect(page).to have_content "VERSION"
|
|
expect(page).to have_content ".gitignore"
|
|
expect(page).to have_content "LICENSE"
|
|
end
|
|
|
|
step 'I should see files from repository for "6d39438"' do
|
|
expect(current_path).to eq namespace_project_tree_path(@project.namespace, @project, "6d39438")
|
|
expect(page).to have_content ".gitignore"
|
|
expect(page).to have_content "LICENSE"
|
|
end
|
|
|
|
step 'I see the ".gitignore"' do
|
|
expect(page).to have_content '.gitignore'
|
|
end
|
|
|
|
step 'I don\'t see the ".gitignore"' do
|
|
expect(page).not_to have_content '.gitignore'
|
|
end
|
|
|
|
step 'I click on ".gitignore" file in repo' do
|
|
click_link ".gitignore"
|
|
end
|
|
|
|
step 'I should see its content' do
|
|
expect(page).to have_content old_gitignore_content
|
|
end
|
|
|
|
step 'I should see its new content' do
|
|
expect(page).to have_content new_gitignore_content
|
|
end
|
|
|
|
step 'I click link "Raw"' do
|
|
click_link 'Raw'
|
|
end
|
|
|
|
step 'I should see raw file content' do
|
|
expect(source).to eq sample_blob.data
|
|
end
|
|
|
|
step 'I click button "Edit"' do
|
|
click_link 'Edit'
|
|
end
|
|
|
|
step 'I cannot see the edit button' do
|
|
expect(page).not_to have_link 'edit'
|
|
end
|
|
|
|
step 'The edit button is disabled' do
|
|
expect(page).to have_css '.disabled', text: 'Edit'
|
|
end
|
|
|
|
step 'I can edit code' do
|
|
set_new_content
|
|
expect(evaluate_script('blob.editor.getValue()')).to eq new_gitignore_content
|
|
end
|
|
|
|
step 'I edit code' do
|
|
set_new_content
|
|
end
|
|
|
|
step 'I fill the new file name' do
|
|
fill_in :file_name, with: new_file_name
|
|
end
|
|
|
|
step 'I fill the new branch name' do
|
|
fill_in :new_branch, with: 'new_branch_name'
|
|
end
|
|
|
|
step 'I fill the new file name with an illegal name' do
|
|
fill_in :file_name, with: 'Spaces Not Allowed'
|
|
end
|
|
|
|
step 'I fill the commit message' do
|
|
fill_in :commit_message, with: 'Not yet a commit message.', visible: true
|
|
end
|
|
|
|
step 'I click link "Diff"' do
|
|
click_link 'Preview changes'
|
|
end
|
|
|
|
step 'I click on "Commit Changes"' do
|
|
click_button 'Commit Changes'
|
|
end
|
|
|
|
step 'I click on "Remove"' do
|
|
click_button 'Remove'
|
|
end
|
|
|
|
step 'I click on "Remove file"' do
|
|
click_button 'Remove file'
|
|
end
|
|
|
|
step 'I click on "Replace"' do
|
|
click_button "Replace"
|
|
end
|
|
|
|
step 'I click on "Replace file"' do
|
|
click_button 'Replace file'
|
|
end
|
|
|
|
step 'I see diff' do
|
|
expect(page).to have_css '.line_holder.new'
|
|
end
|
|
|
|
step 'I click on "new file" link in repo' do
|
|
click_link 'new-file-link'
|
|
end
|
|
|
|
step 'I can see new file page' do
|
|
expect(page).to have_content "new file"
|
|
expect(page).to have_content "Commit message"
|
|
end
|
|
|
|
step 'I can see "upload an existing one"' do
|
|
expect(page).to have_content "upload an existing one"
|
|
end
|
|
|
|
step 'I click on "upload"' do
|
|
click_link 'upload'
|
|
end
|
|
|
|
step 'I click on "Upload file"' do
|
|
click_button 'Upload file'
|
|
end
|
|
|
|
step 'I can see the new commit message' do
|
|
expect(page).to have_content "New upload commit message"
|
|
end
|
|
|
|
step 'I upload a new text file' do
|
|
drop_in_dropzone test_text_file
|
|
end
|
|
|
|
step 'I fill the upload file commit message' do
|
|
page.within('#modal-upload-blob') do
|
|
fill_in :commit_message, with: 'New upload commit message'
|
|
end
|
|
end
|
|
|
|
step 'I replace it with a text file' do
|
|
drop_in_dropzone test_text_file
|
|
end
|
|
|
|
step 'I fill the replace file commit message' do
|
|
page.within('#modal-upload-blob') do
|
|
fill_in :commit_message, with: 'Replacement file commit message'
|
|
end
|
|
end
|
|
|
|
step 'I can see the replacement commit message' do
|
|
expect(page).to have_content "Replacement file commit message"
|
|
end
|
|
|
|
step 'I can see the new text file' do
|
|
expect(page).to have_content "Lorem ipsum dolor sit amet"
|
|
expect(page).to have_content "Sed ut perspiciatis unde omnis"
|
|
end
|
|
|
|
step 'I click on files directory' do
|
|
click_link 'files'
|
|
end
|
|
|
|
step 'I click on History link' do
|
|
click_link 'History'
|
|
end
|
|
|
|
step 'I see Browse dir link' do
|
|
expect(page).to have_link 'Browse Dir »'
|
|
expect(page).not_to have_link 'Browse Code »'
|
|
end
|
|
|
|
step 'I click on readme file' do
|
|
page.within '.tree-table' do
|
|
click_link 'README.md'
|
|
end
|
|
end
|
|
|
|
step 'I see Browse file link' do
|
|
expect(page).to have_link 'Browse File »'
|
|
expect(page).not_to have_link 'Browse Code »'
|
|
end
|
|
|
|
step 'I see Browse code link' do
|
|
expect(page).to have_link 'Browse Code »'
|
|
expect(page).not_to have_link 'Browse File »'
|
|
expect(page).not_to have_link 'Browse Dir »'
|
|
end
|
|
|
|
step 'I click on Permalink' do
|
|
click_link 'Permalink'
|
|
end
|
|
|
|
step 'I am redirected to the files URL' do
|
|
expect(current_path).to eq namespace_project_tree_path(@project.namespace, @project, 'master')
|
|
end
|
|
|
|
step 'I am redirected to the ".gitignore"' do
|
|
expect(current_path).to eq(namespace_project_blob_path(@project.namespace, @project, 'master/.gitignore'))
|
|
end
|
|
|
|
step 'I am redirected to the ".gitignore" on new branch' do
|
|
expect(current_path).to eq(namespace_project_blob_path(@project.namespace, @project, 'new_branch_name/.gitignore'))
|
|
end
|
|
|
|
step 'I am redirected to the permalink URL' do
|
|
expect(current_path).to(
|
|
eq(namespace_project_blob_path(@project.namespace, @project,
|
|
@project.repository.commit.sha +
|
|
'/.gitignore'))
|
|
)
|
|
end
|
|
|
|
step 'I am redirected to the new file' do
|
|
expect(current_path).to eq(namespace_project_blob_path(
|
|
@project.namespace, @project, 'master/' + new_file_name))
|
|
end
|
|
|
|
step 'I am redirected to the new file on new branch' do
|
|
expect(current_path).to eq(namespace_project_blob_path(
|
|
@project.namespace, @project, 'new_branch_name/' + new_file_name))
|
|
end
|
|
|
|
step "I don't see the permalink link" do
|
|
expect(page).not_to have_link('permalink')
|
|
end
|
|
|
|
step 'I see a commit error message' do
|
|
expect(page).to have_content('Your changes could not be committed')
|
|
end
|
|
|
|
step 'I create bare repo' do
|
|
click_link 'Create empty bare repository'
|
|
end
|
|
|
|
step 'I click on "add a file" link' do
|
|
click_link 'adding README'
|
|
|
|
# Remove pre-receive hook so we can push without auth
|
|
FileUtils.rm_f(File.join(@project.repository.path, 'hooks', 'pre-receive'))
|
|
end
|
|
|
|
step "I switch ref to 'test'" do
|
|
select "'test'", from: 'ref'
|
|
end
|
|
|
|
step "I see the ref 'test' has been selected" do
|
|
expect(page).to have_selector '.select2-chosen', text: "'test'"
|
|
end
|
|
|
|
step "I visit the 'test' tree" do
|
|
visit namespace_project_tree_path(@project.namespace, @project, "'test'")
|
|
end
|
|
|
|
step 'I see the commit data' do
|
|
expect(page).to have_css('.tree-commit-link', visible: true)
|
|
expect(page).not_to have_content('Loading commit data...')
|
|
end
|
|
|
|
private
|
|
|
|
def set_new_content
|
|
execute_script("blob.editor.setValue('#{new_gitignore_content}')")
|
|
end
|
|
|
|
# Content of the gitignore file on the seed repository.
|
|
def old_gitignore_content
|
|
'*.rbc'
|
|
end
|
|
|
|
# Constant value that differs from the content
|
|
# of the gitignore of the seed repository.
|
|
def new_gitignore_content
|
|
old_gitignore_content + 'a'
|
|
end
|
|
|
|
# Constant value that is a valid filename and
|
|
# not a filename present at root of the seed repository.
|
|
def new_file_name
|
|
'not_a_file.md'
|
|
end
|
|
|
|
def drop_in_dropzone(file_path)
|
|
# Generate a fake input selector
|
|
page.execute_script <<-JS
|
|
var fakeFileInput = window.$('<input/>').attr(
|
|
{id: 'fakeFileInput', type: 'file'}
|
|
).appendTo('body');
|
|
JS
|
|
# Attach the file to the fake input selector with Capybara
|
|
attach_file("fakeFileInput", file_path)
|
|
# Add the file to a fileList array and trigger the fake drop event
|
|
page.execute_script <<-JS
|
|
var fileList = [$('#fakeFileInput')[0].files[0]];
|
|
var e = jQuery.Event('drop', { dataTransfer : { files : fileList } });
|
|
$('.dropzone')[0].dropzone.listeners[0].events.drop(e);
|
|
JS
|
|
end
|
|
|
|
def test_text_file
|
|
File.join(Rails.root, 'spec', 'fixtures', 'doc_sample.txt')
|
|
end
|
|
|
|
def test_image_file
|
|
File.join(Rails.root, 'spec', 'fixtures', 'banana_sample.gif')
|
|
end
|
|
end
|