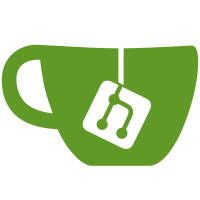
* master: (76 commits) fix failing test fix Vue warnings for missing element [ci skip] UX Guide: Button placement in groups Change window size before visiting page, to get correct scroll position Fix slash commands spec error Move project services to new location under Integrations Move webhooks to new a location under Integrations Fixed redirection from http://someproject.git to http://someproject consistently use single quotes allow application.js to require other scripts which start with application* DRY with Gitlab.config.webpack.dev_server references disable webpack proxy in rspec environment due to conflicts with webmock gem remove changes to Procfile configure webpack dev server port via environment variable add rack middleware to proxy webpack dev server remove dev-server config from development environment Document that the retro and kickoff notes are public add CHAGELOG.md entry for webpack branch fix failing rspec build fix test failure for merge request widget ...
68 lines
2.3 KiB
JavaScript
68 lines
2.3 KiB
JavaScript
/* global gl, Flash */
|
|
/* eslint-disable no-param-reassign, no-underscore-dangle */
|
|
require('../vue_realtime_listener');
|
|
|
|
((gl) => {
|
|
const pageValues = (headers) => {
|
|
const normalized = gl.utils.normalizeHeaders(headers);
|
|
|
|
const paginationInfo = {
|
|
perPage: +normalized['X-PER-PAGE'],
|
|
page: +normalized['X-PAGE'],
|
|
total: +normalized['X-TOTAL'],
|
|
totalPages: +normalized['X-TOTAL-PAGES'],
|
|
nextPage: +normalized['X-NEXT-PAGE'],
|
|
previousPage: +normalized['X-PREV-PAGE'],
|
|
};
|
|
|
|
return paginationInfo;
|
|
};
|
|
|
|
gl.PipelineStore = class {
|
|
fetchDataLoop(Vue, pageNum, url, apiScope) {
|
|
this.pageRequest = true;
|
|
const updatePipelineNums = (count) => {
|
|
const { all } = count;
|
|
const running = count.running_or_pending;
|
|
document.querySelector('.js-totalbuilds-count').innerHTML = all;
|
|
document.querySelector('.js-running-count').innerHTML = running;
|
|
};
|
|
|
|
const goFetch = () =>
|
|
this.$http.get(`${url}?scope=${apiScope}&page=${pageNum}`)
|
|
.then((response) => {
|
|
const pageInfo = pageValues(response.headers);
|
|
this.pageInfo = Object.assign({}, this.pageInfo, pageInfo);
|
|
|
|
const res = JSON.parse(response.body);
|
|
this.count = Object.assign({}, this.count, res.count);
|
|
this.pipelines = Object.assign([], this.pipelines, res.pipelines);
|
|
|
|
updatePipelineNums(this.count);
|
|
this.pageRequest = false;
|
|
}, () => {
|
|
this.pageRequest = false;
|
|
return new Flash('An error occurred while fetching the pipelines, please reload the page again.');
|
|
});
|
|
|
|
goFetch();
|
|
|
|
const startTimeLoops = () => {
|
|
this.timeLoopInterval = setInterval(() => {
|
|
this.$children[0].$children.reduce((acc, component) => {
|
|
const timeAgoComponent = component.$children.filter(el => el.$options._componentTag === 'time-ago')[0];
|
|
acc.push(timeAgoComponent);
|
|
return acc;
|
|
}, []).forEach(e => e.changeTime());
|
|
}, 10000);
|
|
};
|
|
|
|
startTimeLoops();
|
|
|
|
const removeIntervals = () => clearInterval(this.timeLoopInterval);
|
|
const startIntervals = () => startTimeLoops();
|
|
|
|
gl.VueRealtimeListener(removeIntervals, startIntervals);
|
|
}
|
|
};
|
|
})(window.gl || (window.gl = {}));
|