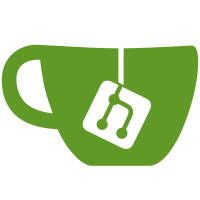
The `access_git` and `access_api` were currently never checked for anonymous users. And they would also be allowed access: An anonymous user can clone and pull from a public repo An anonymous user can request public information from the API So the policy didn't actually reflect what we were enforcing.
183 lines
4.5 KiB
Ruby
183 lines
4.5 KiB
Ruby
require 'spec_helper'
|
|
|
|
describe GlobalPolicy do
|
|
include TermsHelper
|
|
|
|
let(:current_user) { create(:user) }
|
|
let(:user) { create(:user) }
|
|
|
|
subject { described_class.new(current_user, [user]) }
|
|
|
|
describe "reading the list of users" do
|
|
context "for a logged in user" do
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "for an anonymous user" do
|
|
let(:current_user) { nil }
|
|
|
|
context "when the public level is restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [Gitlab::VisibilityLevel::PUBLIC])
|
|
end
|
|
|
|
it { is_expected.not_to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "when the public level is not restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
end
|
|
|
|
context "for an admin" do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
context "when the public level is restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [Gitlab::VisibilityLevel::PUBLIC])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "when the public level is not restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
end
|
|
end
|
|
|
|
describe "create fork" do
|
|
context "when user has not exceeded project limit" do
|
|
it { is_expected.to be_allowed(:create_fork) }
|
|
end
|
|
|
|
context "when user has exceeded project limit" do
|
|
let(:current_user) { create(:user, projects_limit: 0) }
|
|
|
|
it { is_expected.not_to be_allowed(:create_fork) }
|
|
end
|
|
|
|
context "when user is a master in a group" do
|
|
let(:group) { create(:group) }
|
|
let(:current_user) { create(:user, projects_limit: 0) }
|
|
|
|
before do
|
|
group.add_master(current_user)
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:create_fork) }
|
|
end
|
|
end
|
|
|
|
describe 'custom attributes' do
|
|
context 'regular user' do
|
|
it { is_expected.not_to be_allowed(:read_custom_attribute) }
|
|
it { is_expected.not_to be_allowed(:update_custom_attribute) }
|
|
end
|
|
|
|
context 'admin' do
|
|
let(:current_user) { create(:user, :admin) }
|
|
|
|
it { is_expected.to be_allowed(:read_custom_attribute) }
|
|
it { is_expected.to be_allowed(:update_custom_attribute) }
|
|
end
|
|
end
|
|
|
|
shared_examples 'access allowed when terms accepted' do |ability|
|
|
it { is_expected.not_to be_allowed(ability) }
|
|
|
|
it "allows #{ability} when the user accepted the terms" do
|
|
accept_terms(current_user)
|
|
|
|
is_expected.to be_allowed(ability)
|
|
end
|
|
end
|
|
|
|
describe 'API access' do
|
|
context 'regular user' do
|
|
it { is_expected.to be_allowed(:access_api) }
|
|
end
|
|
|
|
context 'admin' do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
it { is_expected.to be_allowed(:access_api) }
|
|
end
|
|
|
|
context 'anonymous' do
|
|
let(:current_user) { nil }
|
|
|
|
it { is_expected.to be_allowed(:access_api) }
|
|
end
|
|
|
|
context 'when terms are enforced' do
|
|
before do
|
|
enforce_terms
|
|
end
|
|
|
|
context 'regular user' do
|
|
it_behaves_like 'access allowed when terms accepted', :access_api
|
|
end
|
|
|
|
context 'admin' do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
it_behaves_like 'access allowed when terms accepted', :access_api
|
|
end
|
|
|
|
context 'anonymous' do
|
|
let(:current_user) { nil }
|
|
|
|
it { is_expected.to be_allowed(:access_api) }
|
|
end
|
|
end
|
|
end
|
|
|
|
describe 'git access' do
|
|
describe 'regular user' do
|
|
it { is_expected.to be_allowed(:access_git) }
|
|
end
|
|
|
|
describe 'admin' do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
it { is_expected.to be_allowed(:access_git) }
|
|
end
|
|
|
|
describe 'anonymous' do
|
|
let(:current_user) { nil }
|
|
|
|
it { is_expected.to be_allowed(:access_git) }
|
|
end
|
|
|
|
context 'when terms are enforced' do
|
|
before do
|
|
enforce_terms
|
|
end
|
|
|
|
context 'regular user' do
|
|
it_behaves_like 'access allowed when terms accepted', :access_git
|
|
end
|
|
|
|
context 'admin' do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
it_behaves_like 'access allowed when terms accepted', :access_git
|
|
end
|
|
|
|
context 'anonymous' do
|
|
let(:current_user) { nil }
|
|
|
|
it { is_expected.to be_allowed(:access_git) }
|
|
end
|
|
end
|
|
end
|
|
end
|