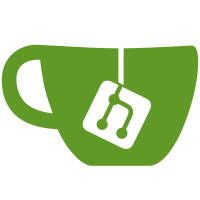
This will be necessary when adding gitaly settings. This version doesn't make any functional changes, but allows us to include this breaking change in 9.0 and add the needed extra settings in the future with backwards compatibility
57 lines
1.9 KiB
Ruby
57 lines
1.9 KiB
Ruby
class PostReceive
|
|
include Sidekiq::Worker
|
|
include DedicatedSidekiqQueue
|
|
|
|
def perform(repo_path, identifier, changes)
|
|
if repository_storage = Gitlab.config.repositories.storages.find { |p| repo_path.start_with?(p[1]['path'].to_s) }
|
|
repo_path.gsub!(repository_storage[1]['path'].to_s, "")
|
|
else
|
|
log("Check gitlab.yml config for correct repositories.storages values. No repository storage path matches \"#{repo_path}\"")
|
|
end
|
|
|
|
changes = Base64.decode64(changes) unless changes.include?(' ')
|
|
# Use Sidekiq.logger so arguments can be correlated with execution
|
|
# time and thread ID's.
|
|
Sidekiq.logger.info "changes: #{changes.inspect}" if ENV['SIDEKIQ_LOG_ARGUMENTS']
|
|
post_received = Gitlab::GitPostReceive.new(repo_path, identifier, changes)
|
|
|
|
if post_received.project.nil?
|
|
log("Triggered hook for non-existing project with full path \"#{repo_path}\"")
|
|
return false
|
|
end
|
|
|
|
if post_received.wiki?
|
|
# Nothing defined here yet.
|
|
elsif post_received.regular_project?
|
|
process_project_changes(post_received)
|
|
else
|
|
log("Triggered hook for unidentifiable repository type with full path \"#{repo_path}\"")
|
|
false
|
|
end
|
|
end
|
|
|
|
def process_project_changes(post_received)
|
|
post_received.changes.each do |change|
|
|
oldrev, newrev, ref = change.strip.split(' ')
|
|
|
|
@user ||= post_received.identify(newrev)
|
|
|
|
unless @user
|
|
log("Triggered hook for non-existing user \"#{post_received.identifier}\"")
|
|
return false
|
|
end
|
|
|
|
if Gitlab::Git.tag_ref?(ref)
|
|
GitTagPushService.new(post_received.project, @user, oldrev: oldrev, newrev: newrev, ref: ref).execute
|
|
elsif Gitlab::Git.branch_ref?(ref)
|
|
GitPushService.new(post_received.project, @user, oldrev: oldrev, newrev: newrev, ref: ref).execute
|
|
end
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def log(message)
|
|
Gitlab::GitLogger.error("POST-RECEIVE: #{message}")
|
|
end
|
|
end
|