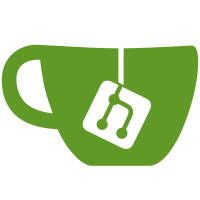
When a restricted visibility level of `private` is set in the instance, creating a snippet with the `visibility` level would always fail. This happened because: 1. `params[:visibility]` was a string (e.g. "public") 2. `CreateSnippetService` and `UpdateSnippetService` only looked at `params[:visibility_level]`, which was `nil`. To fix this, we: 1. Make `CreateSnippetService` look at the newly-built `snippet.visibility_level`, since the right value is assigned by the `VisibilityLevel#visibility=` method. 2. Modify `UpdateSnippetService` to handle both `visibility_level` and `visibility` parameters. Closes https://gitlab.com/gitlab-org/gitlab-ce/issues/66050
32 lines
844 B
Ruby
32 lines
844 B
Ruby
# frozen_string_literal: true
|
|
|
|
class UpdateSnippetService < BaseService
|
|
include SpamCheckService
|
|
|
|
attr_accessor :snippet
|
|
|
|
def initialize(project, user, snippet, params)
|
|
super(project, user, params)
|
|
@snippet = snippet
|
|
end
|
|
|
|
def execute
|
|
# check that user is allowed to set specified visibility_level
|
|
new_visibility = visibility_level
|
|
|
|
if new_visibility && new_visibility.to_i != snippet.visibility_level
|
|
unless Gitlab::VisibilityLevel.allowed_for?(current_user, new_visibility)
|
|
deny_visibility_level(snippet, new_visibility)
|
|
return snippet
|
|
end
|
|
end
|
|
|
|
filter_spam_check_params
|
|
snippet.assign_attributes(params)
|
|
spam_check(snippet, current_user)
|
|
|
|
snippet.save.tap do |succeeded|
|
|
Gitlab::UsageDataCounters::SnippetCounter.count(:update) if succeeded
|
|
end
|
|
end
|
|
end
|