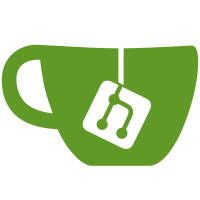
Uses `list_commits_by_oid` on the CommitService, to request the needed commits for pipelines. These commits are needed to display the user that created the commit and the commit title. This includes fixes for tests failing that depended on the commit being `nil`. However, now these are batch loaded, this doesn't happen anymore and the commits are an instance of BatchLoader.
50 lines
1.5 KiB
Ruby
50 lines
1.5 KiB
Ruby
class ExpirePipelineCacheWorker
|
|
include ApplicationWorker
|
|
include PipelineQueue
|
|
|
|
queue_namespace :pipeline_cache
|
|
|
|
def perform(pipeline_id)
|
|
pipeline = Ci::Pipeline.find_by(id: pipeline_id)
|
|
return unless pipeline
|
|
|
|
project = pipeline.project
|
|
store = Gitlab::EtagCaching::Store.new
|
|
|
|
store.touch(project_pipelines_path(project))
|
|
store.touch(project_pipeline_path(project, pipeline))
|
|
store.touch(commit_pipelines_path(project, pipeline.commit)) unless pipeline.commit.nil?
|
|
store.touch(new_merge_request_pipelines_path(project))
|
|
each_pipelines_merge_request_path(project, pipeline) do |path|
|
|
store.touch(path)
|
|
end
|
|
|
|
Gitlab::Cache::Ci::ProjectPipelineStatus.update_for_pipeline(pipeline)
|
|
end
|
|
|
|
private
|
|
|
|
def project_pipelines_path(project)
|
|
Gitlab::Routing.url_helpers.project_pipelines_path(project, format: :json)
|
|
end
|
|
|
|
def project_pipeline_path(project, pipeline)
|
|
Gitlab::Routing.url_helpers.project_pipeline_path(project, pipeline, format: :json)
|
|
end
|
|
|
|
def commit_pipelines_path(project, commit)
|
|
Gitlab::Routing.url_helpers.pipelines_project_commit_path(project, commit.id, format: :json)
|
|
end
|
|
|
|
def new_merge_request_pipelines_path(project)
|
|
Gitlab::Routing.url_helpers.project_new_merge_request_path(project, format: :json)
|
|
end
|
|
|
|
def each_pipelines_merge_request_path(project, pipeline)
|
|
pipeline.all_merge_requests.each do |merge_request|
|
|
path = Gitlab::Routing.url_helpers.pipelines_project_merge_request_path(project, merge_request, format: :json)
|
|
|
|
yield(path)
|
|
end
|
|
end
|
|
end
|