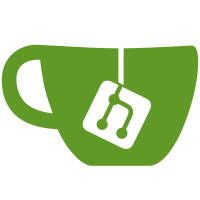
The ApplicationSetting model uses the CacheMarkdownField concern, which updates the cached HTML when the field is updated in the database. However, in specs, when we want to test conditions using ApplicationSetting, we stub it, because this is accessed in different ways throughout the application. This means that if a spec runs that caches one of the Markdown fields, and a later spec uses `stub_application_setting` to set the raw value of that field, the cached value was still the original one. We can work around this by ignoring the Markdown cache in contexts where we're using `stub_application_setting`. We could be smarter, and only do this on the Markdown fields of the model, but this is probably fine.
91 lines
2.8 KiB
Ruby
91 lines
2.8 KiB
Ruby
module StubConfiguration
|
|
def stub_application_setting(messages)
|
|
add_predicates(messages)
|
|
|
|
# Stubbing both of these because we're not yet consistent with how we access
|
|
# current application settings
|
|
allow_any_instance_of(ApplicationSetting).to receive_messages(to_settings(messages))
|
|
allow(Gitlab::CurrentSettings.current_application_settings)
|
|
.to receive_messages(to_settings(messages))
|
|
|
|
# Ensure that we don't use the Markdown cache when stubbing these values
|
|
allow_any_instance_of(ApplicationSetting).to receive(:cached_html_up_to_date?).and_return(false)
|
|
end
|
|
|
|
def stub_not_protect_default_branch
|
|
stub_application_setting(
|
|
default_branch_protection: Gitlab::Access::PROTECTION_NONE)
|
|
end
|
|
|
|
def stub_config_setting(messages)
|
|
allow(Gitlab.config.gitlab).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_gravatar_setting(messages)
|
|
allow(Gitlab.config.gravatar).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_incoming_email_setting(messages)
|
|
allow(Gitlab.config.incoming_email).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_mattermost_setting(messages)
|
|
allow(Gitlab.config.mattermost).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_omniauth_setting(messages)
|
|
allow(Gitlab.config.omniauth).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_backup_setting(messages)
|
|
allow(Gitlab.config.backup).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_lfs_setting(messages)
|
|
allow(Gitlab.config.lfs).to receive_messages(to_settings(messages))
|
|
end
|
|
|
|
def stub_storage_settings(messages)
|
|
messages.deep_stringify_keys!
|
|
|
|
# Default storage is always required
|
|
messages['default'] ||= Gitlab.config.repositories.storages.default
|
|
messages.each do |storage_name, storage_settings|
|
|
storage_settings['path'] = TestEnv.repos_path unless storage_settings.key?('path')
|
|
end
|
|
|
|
allow(Gitlab.config.repositories).to receive(:storages).and_return(Settingslogic.new(messages))
|
|
end
|
|
|
|
private
|
|
|
|
# Modifies stubbed messages to also stub possible predicate versions
|
|
#
|
|
# Examples:
|
|
#
|
|
# add_predicates(foo: true)
|
|
# # => {foo: true, foo?: true}
|
|
#
|
|
# add_predicates(signup_enabled?: false)
|
|
# # => {signup_enabled? false}
|
|
def add_predicates(messages)
|
|
# Only modify keys that aren't already predicates
|
|
keys = messages.keys.map(&:to_s).reject { |k| k.end_with?('?') }
|
|
|
|
keys.each do |key|
|
|
predicate = key + '?'
|
|
messages[predicate.to_sym] = messages[key.to_sym]
|
|
end
|
|
end
|
|
|
|
# Support nested hashes by converting all values into Settingslogic objects
|
|
def to_settings(hash)
|
|
hash.transform_values do |value|
|
|
if value.is_a? Hash
|
|
Settingslogic.new(value.deep_stringify_keys)
|
|
else
|
|
value
|
|
end
|
|
end
|
|
end
|
|
end
|