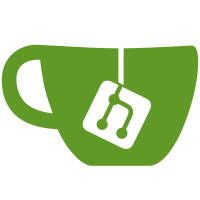
Previously, an HTTP request for `/uploads/-/system/personal_snippet/:snippet_id/:hash/:filename` would look for an uploader of `PersonalFileUploader` class and use `PersonalFileUploader#upload_paths` to search the datbase for one of the following paths: 1. `:hash/:filename` 2. `uploads/-/system/personal_snippet/:id/:hash/:filename` However, when the upload were stored in object storage, `PersonalFileUploader#store_dirs` stored the path as: `personal_snippet/:snippet_id/:hash` The extraneous `uploads/-/system` prefix prevented the path from being matched, and uploads in object storage would return a 404 error. Uploads in local storage would work fine. To fix this, we set the `#base_dir` properly so that `#upload_paths` generates the right value for object storage. Note that this also makes `#store_dirs` do the right thing in `FileUploader`. Closes https://gitlab.com/gitlab-org/gitlab-ce/issues/52595
48 lines
936 B
Ruby
48 lines
936 B
Ruby
# frozen_string_literal: true
|
|
|
|
class PersonalFileUploader < FileUploader
|
|
# Re-Override
|
|
def self.root
|
|
options.storage_path
|
|
end
|
|
|
|
def self.base_dir(model, store = nil)
|
|
base_dirs(model)[store || Store::LOCAL]
|
|
end
|
|
|
|
def self.base_dirs(model)
|
|
{
|
|
Store::LOCAL => File.join(options.base_dir, model_path_segment(model)),
|
|
Store::REMOTE => model_path_segment(model)
|
|
}
|
|
end
|
|
|
|
def self.model_path_segment(model)
|
|
return 'temp/' unless model
|
|
|
|
File.join(model.class.to_s.underscore, model.id.to_s)
|
|
end
|
|
|
|
def object_store
|
|
return Store::LOCAL unless model
|
|
|
|
super
|
|
end
|
|
|
|
# model_path_segment does not require a model to be passed, so we can always
|
|
# generate a path, even when there's no model.
|
|
def model_valid?
|
|
true
|
|
end
|
|
|
|
# Revert-Override
|
|
def store_dir
|
|
store_dirs[object_store]
|
|
end
|
|
|
|
private
|
|
|
|
def secure_url
|
|
File.join('/', base_dir, secret, file.filename)
|
|
end
|
|
end
|