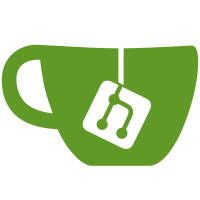
Resolve "Updated UI for Snippets pages" ## What does this MR do? ## Are there points in the code the reviewer needs to double check? ## Why was this MR needed? ## Screenshots (if relevant) ## Does this MR meet the acceptance criteria? - [ ] [Changelog entry](https://docs.gitlab.com/ce/development/changelog.html) added - [ ] [Documentation created/updated](https://gitlab.com/gitlab-org/gitlab-ce/blob/master/doc/development/doc_styleguide.md) - [ ] API support added - Tests - [ ] Added for this feature/bug - [ ] All builds are passing - [ ] Conform by the [merge request performance guides](http://docs.gitlab.com/ce/development/merge_request_performance_guidelines.html) - [ ] Conform by the [style guides](https://gitlab.com/gitlab-org/gitlab-ce/blob/master/CONTRIBUTING.md#style-guides) - [ ] Branch has no merge conflicts with `master` (if it does - rebase it please) - [ ] [Squashed related commits together](https://git-scm.com/book/en/Git-Tools-Rewriting-History#Squashing-Commits) ## What are the relevant issue numbers? Closes #19990 See merge request !7861
66 lines
1.5 KiB
Ruby
66 lines
1.5 KiB
Ruby
class SnippetsFinder
|
|
def execute(current_user, params = {})
|
|
filter = params[:filter]
|
|
user = params.fetch(:user, current_user)
|
|
|
|
case filter
|
|
when :all then
|
|
snippets(current_user).fresh
|
|
when :public then
|
|
Snippet.are_public.fresh
|
|
when :by_user then
|
|
by_user(current_user, user, params[:scope])
|
|
when :by_project
|
|
by_project(current_user, params[:project], params[:scope])
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def snippets(current_user)
|
|
if current_user
|
|
Snippet.public_and_internal
|
|
else
|
|
# Not authenticated
|
|
#
|
|
# Return only:
|
|
# public snippets
|
|
Snippet.are_public
|
|
end
|
|
end
|
|
|
|
def by_user(current_user, user, scope)
|
|
snippets = user.snippets.fresh
|
|
|
|
if current_user
|
|
include_private = user == current_user
|
|
by_scope(snippets, scope, include_private)
|
|
else
|
|
snippets.are_public
|
|
end
|
|
end
|
|
|
|
def by_project(current_user, project, scope)
|
|
snippets = project.snippets.fresh
|
|
|
|
if current_user
|
|
include_private = project.team.member?(current_user) || current_user.admin?
|
|
by_scope(snippets, scope, include_private)
|
|
else
|
|
snippets.are_public
|
|
end
|
|
end
|
|
|
|
def by_scope(snippets, scope = nil, include_private = false)
|
|
case scope.to_s
|
|
when 'are_private'
|
|
include_private ? snippets.are_private : Snippet.none
|
|
when 'are_internal'
|
|
snippets.are_internal
|
|
when 'are_public'
|
|
snippets.are_public
|
|
else
|
|
include_private ? snippets : snippets.public_and_internal
|
|
end
|
|
end
|
|
end
|