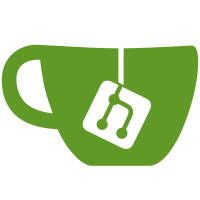
This enforces the terms in the web application. These cases are specced: - Logging in: When terms are enforced, and a user logs in that has not accepted the terms, they are presented with the screen. They get directed to their customized root path afterwards. - Signing up: After signing up, the first screen the user is presented with the screen to accept the terms. After they accept they are directed to the dashboard. - While a session is active: - For a GET: The user will be directed to the terms page first, after they accept the terms, they will be directed to the page they were going to - For any other request: They are directed to the terms, after they accept the terms, they are directed back to the page they came from to retry the request. Any information entered would be persisted in localstorage and available on the page.
93 lines
2.5 KiB
Ruby
93 lines
2.5 KiB
Ruby
require 'spec_helper'
|
|
|
|
describe GlobalPolicy do
|
|
include TermsHelper
|
|
|
|
let(:current_user) { create(:user) }
|
|
let(:user) { create(:user) }
|
|
|
|
subject { described_class.new(current_user, [user]) }
|
|
|
|
describe "reading the list of users" do
|
|
context "for a logged in user" do
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "for an anonymous user" do
|
|
let(:current_user) { nil }
|
|
|
|
context "when the public level is restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [Gitlab::VisibilityLevel::PUBLIC])
|
|
end
|
|
|
|
it { is_expected.not_to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "when the public level is not restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
end
|
|
|
|
context "for an admin" do
|
|
let(:current_user) { create(:admin) }
|
|
|
|
context "when the public level is restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [Gitlab::VisibilityLevel::PUBLIC])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
|
|
context "when the public level is not restricted" do
|
|
before do
|
|
stub_application_setting(restricted_visibility_levels: [])
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:read_users_list) }
|
|
end
|
|
end
|
|
end
|
|
|
|
describe "create fork" do
|
|
context "when user has not exceeded project limit" do
|
|
it { is_expected.to be_allowed(:create_fork) }
|
|
end
|
|
|
|
context "when user has exceeded project limit" do
|
|
let(:current_user) { create(:user, projects_limit: 0) }
|
|
|
|
it { is_expected.not_to be_allowed(:create_fork) }
|
|
end
|
|
|
|
context "when user is a master in a group" do
|
|
let(:group) { create(:group) }
|
|
let(:current_user) { create(:user, projects_limit: 0) }
|
|
|
|
before do
|
|
group.add_master(current_user)
|
|
end
|
|
|
|
it { is_expected.to be_allowed(:create_fork) }
|
|
end
|
|
end
|
|
|
|
describe 'custom attributes' do
|
|
context 'regular user' do
|
|
it { is_expected.not_to be_allowed(:read_custom_attribute) }
|
|
it { is_expected.not_to be_allowed(:update_custom_attribute) }
|
|
end
|
|
|
|
context 'admin' do
|
|
let(:current_user) { create(:user, :admin) }
|
|
|
|
it { is_expected.to be_allowed(:read_custom_attribute) }
|
|
it { is_expected.to be_allowed(:update_custom_attribute) }
|
|
end
|
|
end
|
|
end
|