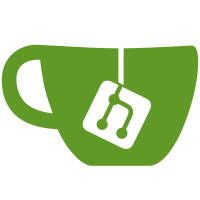
- Have `Uniquify` take a block instead of a Proc/function. This is more idiomatic than passing around a function in Ruby. - Block a user before moving their issues to the ghost user. This avoids a data race where an issue is created after the issues are migrated to the ghost user, and before the destroy takes place. - No need to migrate issues (to the ghost user) in a transaction, because we're using `update_all` - Other minor changes
30 lines
645 B
Ruby
30 lines
645 B
Ruby
class Uniquify
|
|
# Return a version of the given 'base' string that is unique
|
|
# by appending a counter to it. Uniqueness is determined by
|
|
# repeated calls to the passed block.
|
|
#
|
|
# If `base` is a function/proc, we expect that calling it with a
|
|
# candidate counter returns a string to test/return.
|
|
def string(base)
|
|
@base = base
|
|
@counter = nil
|
|
|
|
increment_counter! while yield(base_string)
|
|
base_string
|
|
end
|
|
|
|
private
|
|
|
|
def base_string
|
|
if @base.respond_to?(:call)
|
|
@base.call(@counter)
|
|
else
|
|
"#{@base}#{@counter}"
|
|
end
|
|
end
|
|
|
|
def increment_counter!
|
|
@counter ||= 0
|
|
@counter += 1
|
|
end
|
|
end
|