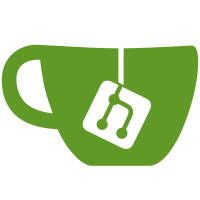
This adds the 2016 emoji as well as support for using SVG images instead of PNGs. It also fixes a number of incorrectly categorized emoji and other minor issues. Upgrade Rake task for Gemojione 3.0.0 and generate sprites. Upgrade aliases.json by pulling down index.json from the gemojione repository and running the generate_aliases.rb file. Changelog: https://github.com/jonathanwiesel/gemojione/blob/master/CHANGELOG.md#v301-2016-07-16 For the specific emoji added to the Unicode standard, see: http://emojione.com/releases/2.2.4/ Huge kudos to Jonathan Wiesel (@jonathanwiesel) for his work on the gemojione gem!
83 lines
2.5 KiB
Ruby
83 lines
2.5 KiB
Ruby
module Gitlab
|
|
class AwardEmoji
|
|
CATEGORIES = {
|
|
objects: "Objects",
|
|
travel: "Travel",
|
|
symbols: "Symbols",
|
|
nature: "Nature",
|
|
people: "People",
|
|
activity: "Activity",
|
|
flags: "Flags",
|
|
food: "Food"
|
|
}.with_indifferent_access
|
|
|
|
def self.normalize_emoji_name(name)
|
|
aliases[name] || name
|
|
end
|
|
|
|
def self.emoji_by_category
|
|
unless @emoji_by_category
|
|
@emoji_by_category = Hash.new { |h, key| h[key] = [] }
|
|
|
|
emojis.each do |emoji_name, data|
|
|
data["name"] = emoji_name
|
|
|
|
# Skip Fitzpatrick(tone) modifiers
|
|
next if data["category"] == "modifier"
|
|
|
|
category = data["category"]
|
|
|
|
@emoji_by_category[category] << data
|
|
end
|
|
|
|
@emoji_by_category = @emoji_by_category.sort.to_h
|
|
end
|
|
|
|
@emoji_by_category
|
|
end
|
|
|
|
def self.emojis
|
|
@emojis ||=
|
|
begin
|
|
json_path = File.join(Rails.root, 'fixtures', 'emojis', 'index.json' )
|
|
JSON.parse(File.read(json_path))
|
|
end
|
|
end
|
|
|
|
def self.aliases
|
|
@aliases ||=
|
|
begin
|
|
json_path = File.join(Rails.root, 'fixtures', 'emojis', 'aliases.json')
|
|
JSON.parse(File.read(json_path))
|
|
end
|
|
end
|
|
|
|
# Returns an Array of Emoji names and their asset URLs.
|
|
def self.urls
|
|
@urls ||= begin
|
|
path = File.join(Rails.root, 'fixtures', 'emojis', 'digests.json')
|
|
# Construct the full asset path ourselves because
|
|
# ActionView::Helpers::AssetUrlHelper.asset_url is slow for hundreds
|
|
# of entries since it has to do a lot of extra work (e.g. regexps).
|
|
prefix = Gitlab::Application.config.assets.prefix
|
|
digest = Gitlab::Application.config.assets.digest
|
|
base =
|
|
if defined?(Gitlab::Application.config.relative_url_root) && Gitlab::Application.config.relative_url_root
|
|
Gitlab::Application.config.relative_url_root
|
|
else
|
|
''
|
|
end
|
|
|
|
JSON.parse(File.read(path)).map do |hash|
|
|
if digest
|
|
fname = "#{hash['unicode']}-#{hash['digest']}"
|
|
else
|
|
fname = hash['unicode']
|
|
end
|
|
|
|
{ name: hash['name'], path: File.join(base, prefix, "#{fname}.png") }
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|