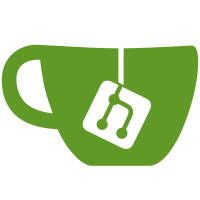
This sets up all the basics for importing Phabricator tasks into GitLab issues. To import all tasks from a Phabricator instance into GitLab, we'll import all of them into a new project that will have its repository disabled. The import is hooked into a regular ProjectImport setup, but similar to the GitHub parallel importer takes care of all the imports itself. In this iteration, we're importing each page of tasks in a separate sidekiq job. The first thing we do when requesting a new page of tasks is schedule the next page to be imported. But to avoid deadlocks, we only allow a single job per worker type to run at the same time. For now we're only importing basic Issue information, this should be extended to richer information.
92 lines
2.5 KiB
Ruby
92 lines
2.5 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require 'spec_helper'
|
|
|
|
describe Import::PhabricatorController do
|
|
let(:current_user) { create(:user) }
|
|
|
|
before do
|
|
sign_in current_user
|
|
end
|
|
|
|
describe 'GET #new' do
|
|
subject { get :new }
|
|
|
|
context 'when the import source is not available' do
|
|
before do
|
|
stub_feature_flags(phabricator_import: true)
|
|
stub_application_setting(import_sources: [])
|
|
end
|
|
|
|
it { is_expected.to have_gitlab_http_status(404) }
|
|
end
|
|
|
|
context 'when the feature is disabled' do
|
|
before do
|
|
stub_feature_flags(phabricator_import: false)
|
|
stub_application_setting(import_sources: ['phabricator'])
|
|
end
|
|
|
|
it { is_expected.to have_gitlab_http_status(404) }
|
|
end
|
|
|
|
context 'when the import is available' do
|
|
before do
|
|
stub_feature_flags(phabricator_import: true)
|
|
stub_application_setting(import_sources: ['phabricator'])
|
|
end
|
|
|
|
it { is_expected.to have_gitlab_http_status(200) }
|
|
end
|
|
end
|
|
|
|
describe 'POST #create' do
|
|
subject(:post_create) { post :create, params: params }
|
|
|
|
context 'with valid params' do
|
|
let(:params) do
|
|
{ path: 'phab-import',
|
|
name: 'Phab import',
|
|
phabricator_server_url: 'https://phabricator.example.com',
|
|
api_token: 'hazaah',
|
|
namespace_id: current_user.namespace_id }
|
|
end
|
|
|
|
it 'creates a project to import' do
|
|
expect_next_instance_of(Gitlab::PhabricatorImport::Importer) do |importer|
|
|
expect(importer).to receive(:execute)
|
|
end
|
|
|
|
expect { post_create }.to change { current_user.namespace.projects.reload.size }.from(0).to(1)
|
|
|
|
expect(current_user.namespace.projects.last).to be_import
|
|
end
|
|
end
|
|
|
|
context 'when an import param is missing' do
|
|
let(:params) do
|
|
{ path: 'phab-import',
|
|
name: 'Phab import',
|
|
phabricator_server_url: nil,
|
|
api_token: 'hazaah',
|
|
namespace_id: current_user.namespace_id }
|
|
end
|
|
|
|
it 'does not create the project' do
|
|
expect { post_create }.not_to change { current_user.namespace.projects.reload.size }
|
|
end
|
|
end
|
|
|
|
context 'when a project param is missing' do
|
|
let(:params) do
|
|
{ phabricator_server_url: 'https://phabricator.example.com',
|
|
api_token: 'hazaah',
|
|
namespace_id: current_user.namespace_id }
|
|
end
|
|
|
|
it 'does not create the project' do
|
|
expect { post_create }.not_to change { current_user.namespace.projects.reload.size }
|
|
end
|
|
end
|
|
end
|
|
end
|