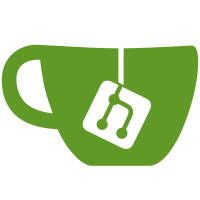
This commit includes a couple of thing: - A chatops controller - Mattermost::CommandService - Mattermost::Commands::(IssueService|MergeRequestService) The controller is the point where mattermost, and later slack will have to fire their payload to. This in turn will execute the CommandService. Thats where the authentication and authorization should happen. So far this is not yet implemented. This should happen in later commits. Per subcommand, in case of `/gitlab issue show 123` issue whould be the subcommand, there is a service to parse the data, and fetch the resource. The resource is passed back to the CommandService which structures the data.
93 lines
2.8 KiB
Ruby
93 lines
2.8 KiB
Ruby
class ProjectFeature < ActiveRecord::Base
|
|
# == Project features permissions
|
|
#
|
|
# Grants access level to project tools
|
|
#
|
|
# Tools can be enabled only for users, everyone or disabled
|
|
# Access control is made only for non private projects
|
|
#
|
|
# levels:
|
|
#
|
|
# Disabled: not enabled for anyone
|
|
# Private: enabled only for team members
|
|
# Enabled: enabled for everyone able to access the project
|
|
#
|
|
|
|
# Permission levels
|
|
DISABLED = 0
|
|
PRIVATE = 10
|
|
ENABLED = 20
|
|
|
|
FEATURES = %i(issues merge_requests wiki snippets builds repository)
|
|
|
|
class << self
|
|
def access_level_attribute(feature)
|
|
feature = feature.model_name.plural.to_sym if feature.respond_to?(:model_name)
|
|
raise ArgumentError, "invalid project feature: #{feature}" unless FEATURES.include?(feature)
|
|
|
|
"#{feature}_access_level".to_sym
|
|
end
|
|
end
|
|
|
|
# Default scopes force us to unscope here since a service may need to check
|
|
# permissions for a project in pending_delete
|
|
# http://stackoverflow.com/questions/1540645/how-to-disable-default-scope-for-a-belongs-to
|
|
belongs_to :project, -> { unscope(where: :pending_delete) }
|
|
|
|
validate :repository_children_level
|
|
|
|
default_value_for :builds_access_level, value: ENABLED, allows_nil: false
|
|
default_value_for :issues_access_level, value: ENABLED, allows_nil: false
|
|
default_value_for :merge_requests_access_level, value: ENABLED, allows_nil: false
|
|
default_value_for :snippets_access_level, value: ENABLED, allows_nil: false
|
|
default_value_for :wiki_access_level, value: ENABLED, allows_nil: false
|
|
default_value_for :repository_access_level, value: ENABLED, allows_nil: false
|
|
|
|
def feature_available?(feature, user)
|
|
access_level = public_send(ProjectFeature.access_level_attribute(feature))
|
|
get_permission(user, access_level)
|
|
end
|
|
|
|
def builds_enabled?
|
|
builds_access_level > DISABLED
|
|
end
|
|
|
|
def wiki_enabled?
|
|
wiki_access_level > DISABLED
|
|
end
|
|
|
|
def merge_requests_enabled?
|
|
merge_requests_access_level > DISABLED
|
|
end
|
|
|
|
def issues_enabled?
|
|
issues_access_level > DISABLED
|
|
end
|
|
|
|
private
|
|
|
|
# Validates builds and merge requests access level
|
|
# which cannot be higher than repository access level
|
|
def repository_children_level
|
|
validator = lambda do |field|
|
|
level = public_send(field) || ProjectFeature::ENABLED
|
|
not_allowed = level > repository_access_level
|
|
self.errors.add(field, "cannot have higher visibility level than repository access level") if not_allowed
|
|
end
|
|
|
|
%i(merge_requests_access_level builds_access_level).each(&validator)
|
|
end
|
|
|
|
def get_permission(user, level)
|
|
case level
|
|
when DISABLED
|
|
false
|
|
when PRIVATE
|
|
user && (project.team.member?(user) || user.admin?)
|
|
when ENABLED
|
|
true
|
|
else
|
|
true
|
|
end
|
|
end
|
|
end
|