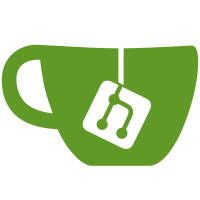
This commit adds the module `FromUnion`, which provides the class method `from_union`. This simplifies the process of selecting data from the result of a UNION, and reduces the likelihood of making mistakes. As a result, instead of this: union = Gitlab::SQL::Union.new([foo, bar]) Foo.from("(#{union.to_sql}) #{Foo.table_name}") We can now write this instead: Foo.from_union([foo, bar]) This commit also includes some changes to make this new setup work properly. For example, a bug in Rails 4 (https://github.com/rails/rails/issues/24193) would break the use of `from("sub-query-here").includes(:relation)` in certain cases. There was also a CI query which appeared to repeat a lot of conditions from an outer query on an inner query, which isn't necessary. Finally, we include a RuboCop cop to ensure developers use this new module, instead of using Gitlab::SQL::Union directly. Fixes https://gitlab.com/gitlab-org/gitlab-ce/issues/51307
40 lines
1,006 B
Ruby
40 lines
1,006 B
Ruby
# frozen_string_literal: true
|
|
|
|
require 'spec_helper'
|
|
|
|
describe FromUnion do
|
|
describe '.from_union' do
|
|
let(:model) do
|
|
Class.new(ActiveRecord::Base) do
|
|
self.table_name = 'users'
|
|
|
|
include FromUnion
|
|
end
|
|
end
|
|
|
|
it 'selects from the results of the UNION' do
|
|
query = model.from_union([model.where(id: 1), model.where(id: 2)])
|
|
|
|
expect(query.to_sql).to match(/FROM \(SELECT.+UNION.+SELECT.+\) users/m)
|
|
end
|
|
|
|
it 'supports the use of a custom alias for the sub query' do
|
|
query = model.from_union(
|
|
[model.where(id: 1), model.where(id: 2)],
|
|
alias_as: 'kittens'
|
|
)
|
|
|
|
expect(query.to_sql).to match(/FROM \(SELECT.+UNION.+SELECT.+\) kittens/m)
|
|
end
|
|
|
|
it 'supports keeping duplicate rows' do
|
|
query = model.from_union(
|
|
[model.where(id: 1), model.where(id: 2)],
|
|
remove_duplicates: false
|
|
)
|
|
|
|
expect(query.to_sql)
|
|
.to match(/FROM \(SELECT.+UNION ALL.+SELECT.+\) users/m)
|
|
end
|
|
end
|
|
end
|