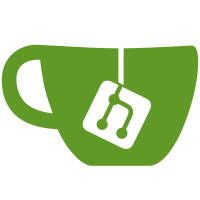
* master: (314 commits) Better Explore Groups view Update Carrierwave and fog-core Add specs for Gitlab::RequestProfiler Add scripts/static-analysis to run all the static analysers in one go Shorten and improve some job names Group static-analysis jobs into a single job Don't blow up when email has no References header Update CHANGELOG.md for 9.1.2 Add changelog Add changelog Show Raw button as Download for binary files Use blob viewers for snippets Fix typo Fixed transient failure related to dropdown animations Revert "Merge branch 'tc-no-todo-service-select' into 'master'" fix link to MR 10416 Another change from .click -> .trigger('click') to make spec pass Change from .click -> .trigger('click') to make spec pass Disable AddColumnWithDefaultToLargeTable cop for pre-existing migrations Add AddColumnWithDefaultToLargeTable cop ... Conflicts: spec/requests/api/jobs_spec.rb
243 lines
7.6 KiB
Ruby
243 lines
7.6 KiB
Ruby
require 'spec_helper'
|
|
|
|
feature 'Environment', :feature do
|
|
given(:project) { create(:empty_project) }
|
|
given(:user) { create(:user) }
|
|
given(:role) { :developer }
|
|
|
|
background do
|
|
login_as(user)
|
|
project.team << [user, role]
|
|
end
|
|
|
|
feature 'environment details page' do
|
|
given!(:environment) { create(:environment, project: project) }
|
|
given!(:deployment) { }
|
|
given!(:action) { }
|
|
|
|
before do
|
|
visit_environment(environment)
|
|
end
|
|
|
|
scenario 'shows environment name' do
|
|
expect(page).to have_content(environment.name)
|
|
end
|
|
|
|
context 'without deployments' do
|
|
scenario 'does show no deployments' do
|
|
expect(page).to have_content('You don\'t have any deployments right now.')
|
|
end
|
|
end
|
|
|
|
context 'with deployments' do
|
|
context 'when there is no related deployable' do
|
|
given(:deployment) do
|
|
create(:deployment, environment: environment, deployable: nil)
|
|
end
|
|
|
|
scenario 'does show deployment SHA' do
|
|
expect(page).to have_link(deployment.short_sha)
|
|
expect(page).not_to have_link('Re-deploy')
|
|
expect(page).not_to have_terminal_button
|
|
end
|
|
end
|
|
|
|
context 'with related deployable present' do
|
|
given(:pipeline) { create(:ci_pipeline, project: project) }
|
|
given(:build) { create(:ci_build, pipeline: pipeline) }
|
|
|
|
given(:deployment) do
|
|
create(:deployment, environment: environment, deployable: build)
|
|
end
|
|
|
|
scenario 'does show build name' do
|
|
expect(page).to have_link("#{build.name} (##{build.id})")
|
|
expect(page).to have_link('Re-deploy')
|
|
expect(page).not_to have_terminal_button
|
|
end
|
|
|
|
context 'with manual action' do
|
|
given(:action) do
|
|
create(:ci_build, :manual, pipeline: pipeline,
|
|
name: 'deploy to production')
|
|
end
|
|
|
|
given(:role) { :master }
|
|
|
|
scenario 'does show a play button' do
|
|
expect(page).to have_link(action.name.humanize)
|
|
end
|
|
|
|
scenario 'does allow to play manual action' do
|
|
expect(action).to be_manual
|
|
|
|
expect { click_link(action.name.humanize) }
|
|
.not_to change { Ci::Pipeline.count }
|
|
|
|
expect(page).to have_content(action.name)
|
|
expect(action.reload).to be_pending
|
|
end
|
|
|
|
context 'with external_url' do
|
|
given(:environment) { create(:environment, project: project, external_url: 'https://git.gitlab.com') }
|
|
given(:build) { create(:ci_build, pipeline: pipeline) }
|
|
given(:deployment) { create(:deployment, environment: environment, deployable: build) }
|
|
|
|
scenario 'does show an external link button' do
|
|
expect(page).to have_link(nil, href: environment.external_url)
|
|
end
|
|
end
|
|
|
|
context 'with terminal' do
|
|
let(:project) { create(:kubernetes_project, :test_repo) }
|
|
|
|
context 'for project master' do
|
|
let(:role) { :master }
|
|
|
|
scenario 'it shows the terminal button' do
|
|
expect(page).to have_terminal_button
|
|
end
|
|
|
|
context 'web terminal', :js do
|
|
before do
|
|
# Stub #terminals as it causes js-enabled feature specs to render the page incorrectly
|
|
allow_any_instance_of(Environment).to receive(:terminals) { nil }
|
|
visit terminal_namespace_project_environment_path(project.namespace, project, environment)
|
|
end
|
|
|
|
it 'displays a web terminal' do
|
|
expect(page).to have_selector('#terminal')
|
|
expect(page).to have_link(nil, href: environment.external_url)
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'for developer' do
|
|
let(:role) { :developer }
|
|
|
|
scenario 'does not show terminal button' do
|
|
expect(page).not_to have_terminal_button
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'when environment is available' do
|
|
context 'with stop action' do
|
|
given(:action) do
|
|
create(:ci_build, :manual, pipeline: pipeline,
|
|
name: 'close_app')
|
|
end
|
|
|
|
given(:deployment) do
|
|
create(:deployment, environment: environment,
|
|
deployable: build,
|
|
on_stop: 'close_app')
|
|
end
|
|
|
|
given(:role) { :master }
|
|
|
|
scenario 'does allow to stop environment' do
|
|
click_link('Stop')
|
|
|
|
expect(page).to have_content('close_app')
|
|
end
|
|
|
|
context 'for reporter' do
|
|
let(:role) { :reporter }
|
|
|
|
scenario 'does not show stop button' do
|
|
expect(page).not_to have_link('Stop')
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'without stop action' do
|
|
scenario 'does allow to stop environment' do
|
|
click_link('Stop')
|
|
end
|
|
end
|
|
end
|
|
|
|
context 'when environment is stopped' do
|
|
given(:environment) { create(:environment, project: project, state: :stopped) }
|
|
|
|
scenario 'does not show stop button' do
|
|
expect(page).not_to have_link('Stop')
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
feature 'environment folders', :js do
|
|
context 'when folder name contains special charaters' do
|
|
before do
|
|
create(:environment, project: project,
|
|
name: 'staging-1.0/review',
|
|
state: :available)
|
|
|
|
visit folder_namespace_project_environments_path(project.namespace,
|
|
project,
|
|
id: 'staging-1.0')
|
|
end
|
|
|
|
it 'renders a correct environment folder' do
|
|
expect(page).to have_http_status(:ok)
|
|
expect(page).to have_content('Environments / staging-1.0')
|
|
end
|
|
end
|
|
end
|
|
|
|
feature 'auto-close environment when branch is deleted' do
|
|
given(:project) { create(:project) }
|
|
|
|
given!(:environment) do
|
|
create(:environment, :with_review_app, project: project,
|
|
ref: 'feature')
|
|
end
|
|
|
|
scenario 'user visits environment page' do
|
|
visit_environment(environment)
|
|
|
|
expect(page).to have_link('Stop')
|
|
end
|
|
|
|
scenario 'user deletes the branch with running environment' do
|
|
visit namespace_project_branches_path(project.namespace, project, search: 'feature')
|
|
|
|
remove_branch_with_hooks(project, user, 'feature') do
|
|
page.within('.js-branch-feature') { find('a.btn-remove').click }
|
|
end
|
|
|
|
visit_environment(environment)
|
|
|
|
expect(page).to have_no_link('Stop')
|
|
end
|
|
|
|
##
|
|
# This is a workaround for problem described in #24543
|
|
#
|
|
def remove_branch_with_hooks(project, user, branch)
|
|
params = {
|
|
oldrev: project.commit(branch).id,
|
|
newrev: Gitlab::Git::BLANK_SHA,
|
|
ref: "refs/heads/#{branch}"
|
|
}
|
|
|
|
yield
|
|
|
|
GitPushService.new(project, user, params).execute
|
|
end
|
|
end
|
|
|
|
def visit_environment(environment)
|
|
visit namespace_project_environment_path(environment.project.namespace,
|
|
environment.project,
|
|
environment)
|
|
end
|
|
|
|
def have_terminal_button
|
|
have_link(nil, href: terminal_namespace_project_environment_path(project.namespace, project, environment))
|
|
end
|
|
end
|