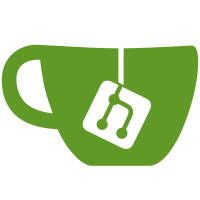
Prevents refspec as branch name, which would bypass branch protection when used in conjunction with rebase. HEAD seems to be a special case with lots of occurrence, so it is considered valid for now. Another special case is `refs/head/*`, which can be imported.
42 lines
1.1 KiB
Ruby
42 lines
1.1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
# Gitaly note: JV: does not need to be migrated, works without a repo.
|
|
|
|
module Gitlab
|
|
module GitRefValidator
|
|
extend self
|
|
|
|
EXPANDED_PREFIXES = %w[refs/heads/ refs/remotes/].freeze
|
|
DISALLOWED_PREFIXES = %w[-].freeze
|
|
|
|
# Validates a given name against the git reference specification
|
|
#
|
|
# Returns true for a valid reference name, false otherwise
|
|
def validate(ref_name)
|
|
return false if ref_name.start_with?(*(EXPANDED_PREFIXES + DISALLOWED_PREFIXES))
|
|
return false if ref_name == 'HEAD'
|
|
|
|
begin
|
|
Rugged::Reference.valid_name?("refs/heads/#{ref_name}")
|
|
rescue ArgumentError
|
|
return false
|
|
end
|
|
end
|
|
|
|
def validate_merge_request_branch(ref_name)
|
|
return false if ref_name.start_with?(*DISALLOWED_PREFIXES)
|
|
|
|
expanded_name = if ref_name.start_with?(*EXPANDED_PREFIXES)
|
|
ref_name
|
|
else
|
|
"refs/heads/#{ref_name}"
|
|
end
|
|
|
|
begin
|
|
Rugged::Reference.valid_name?(expanded_name)
|
|
rescue ArgumentError
|
|
return false
|
|
end
|
|
end
|
|
end
|
|
end
|