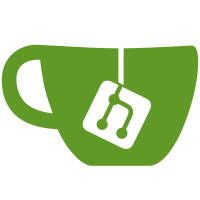
This ensures that we have more visibility in the number of SQL queries that are executed in web requests. The current threshold is hardcoded to 100 as we will rarely (maybe once or twice) change it. In production and development we use Sentry if enabled, in the test environment we raise an error. This feature is also only enabled in production/staging when running on GitLab.com as it's not very useful to other users.
73 lines
2.2 KiB
Ruby
73 lines
2.2 KiB
Ruby
require "base64"
|
|
|
|
class Projects::CommitsController < Projects::ApplicationController
|
|
include ExtractsPath
|
|
include RendersCommits
|
|
|
|
before_action :whitelist_query_limiting
|
|
before_action :require_non_empty_project
|
|
before_action :assign_ref_vars
|
|
before_action :authorize_download_code!
|
|
before_action :set_commits
|
|
|
|
def show
|
|
@merge_request = MergeRequestsFinder.new(current_user, project_id: @project.id).execute.opened
|
|
.find_by(source_project: @project, source_branch: @ref, target_branch: @repository.root_ref)
|
|
|
|
# https://gitlab.com/gitlab-org/gitaly/issues/931
|
|
Gitlab::GitalyClient.allow_n_plus_1_calls do
|
|
respond_to do |format|
|
|
format.html
|
|
format.atom { render layout: 'xml.atom' }
|
|
|
|
format.json do
|
|
pager_json(
|
|
'projects/commits/_commits',
|
|
@commits.size,
|
|
project: @project,
|
|
ref: @ref)
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
def signatures
|
|
# https://gitlab.com/gitlab-org/gitaly/issues/931
|
|
Gitlab::GitalyClient.allow_n_plus_1_calls do
|
|
respond_to do |format|
|
|
format.json do
|
|
render json: {
|
|
signatures: @commits.select(&:has_signature?).map do |commit|
|
|
{
|
|
commit_sha: commit.sha,
|
|
html: view_to_html_string('projects/commit/_signature', signature: commit.signature)
|
|
}
|
|
end
|
|
}
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def set_commits
|
|
render_404 unless @path.empty? || request.format == :atom || @repository.blob_at(@commit.id, @path) || @repository.tree(@commit.id, @path).entries.present?
|
|
@limit, @offset = (params[:limit] || 40).to_i, (params[:offset] || 0).to_i
|
|
search = params[:search]
|
|
|
|
@commits =
|
|
if search.present?
|
|
@repository.find_commits_by_message(search, @ref, @path, @limit, @offset)
|
|
else
|
|
@repository.commits(@ref, path: @path, limit: @limit, offset: @offset)
|
|
end
|
|
|
|
@commits = @commits.with_pipeline_status
|
|
@commits = prepare_commits_for_rendering(@commits)
|
|
end
|
|
|
|
def whitelist_query_limiting
|
|
Gitlab::QueryLimiting.whitelist('https://gitlab.com/gitlab-org/gitlab-ce/issues/42330')
|
|
end
|
|
end
|