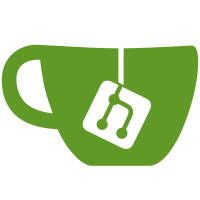
This helper is used for extracting part of the issue / MR / whatever description for use in the description meta tag: 1. To do that, we look at the source of the Markdown description. 2. We then strip out all HTML tags. 3. And then take the first 30 words. Doing that can be really slow - especially as Markdown is supposed to be treated as plain text. There are many better ways to do this, but the immediate performance fix is to swap steps 2 and 3. This does mean that the description may be less than 30 words (or even empty), but it is much faster when the description is very long.
118 lines
3.7 KiB
Ruby
118 lines
3.7 KiB
Ruby
require 'rails_helper'
|
|
|
|
describe PageLayoutHelper do
|
|
describe 'page_description' do
|
|
it 'defaults to nil' do
|
|
expect(helper.page_description).to eq nil
|
|
end
|
|
|
|
it 'returns the last-pushed description' do
|
|
helper.page_description('Foo')
|
|
helper.page_description('Bar')
|
|
helper.page_description('Baz')
|
|
|
|
expect(helper.page_description).to eq 'Baz'
|
|
end
|
|
|
|
it 'squishes multiple newlines' do
|
|
helper.page_description("Foo\nBar\nBaz")
|
|
|
|
expect(helper.page_description).to eq 'Foo Bar Baz'
|
|
end
|
|
|
|
it 'truncates' do
|
|
helper.page_description <<-LOREM.strip_heredoc
|
|
Lorem ipsum dolor sit amet, consectetuer adipiscing elit. Aenean commodo
|
|
ligula eget dolor. Aenean massa. Cum sociis natoque penatibus et magnis
|
|
dis parturient montes, nascetur ridiculus mus. Donec quam felis,
|
|
ultricies nec, pellentesque eu, pretium quis, sem. Nulla consequat massa
|
|
quis enim. Donec pede justo, fringilla vel, aliquet nec, vulputate eget,
|
|
arcu.
|
|
LOREM
|
|
|
|
expect(helper.page_description).to end_with 'quam felis,...'
|
|
end
|
|
|
|
it 'sanitizes all HTML' do
|
|
helper.page_description("<b>Bold</b> <h1>Header</h1>")
|
|
|
|
expect(helper.page_description).to eq 'Bold Header'
|
|
end
|
|
|
|
it 'truncates before sanitizing' do
|
|
helper.page_description('<b>Bold</b> <img> <img> <img> <h1>Header</h1> ' * 10)
|
|
|
|
# 12 words because <img> was counted as a word
|
|
expect(helper.page_description)
|
|
.to eq('Bold Header Bold Header Bold Header Bold Header Bold Header Bold Header...')
|
|
end
|
|
end
|
|
|
|
describe 'page_image' do
|
|
it 'defaults to the GitLab logo' do
|
|
expect(helper.page_image).to match_asset_path 'assets/gitlab_logo.png'
|
|
end
|
|
|
|
%w(project user group).each do |type|
|
|
context "with @#{type} assigned" do
|
|
it "uses #{type.titlecase} avatar if available" do
|
|
object = double(avatar_url: 'http://example.com/uploads/-/system/avatar.png')
|
|
assign(type, object)
|
|
|
|
expect(helper.page_image).to eq object.avatar_url
|
|
end
|
|
|
|
it 'falls back to the default when avatar_url is nil' do
|
|
object = double(avatar_url: nil)
|
|
assign(type, object)
|
|
|
|
expect(helper.page_image).to match_asset_path 'assets/gitlab_logo.png'
|
|
end
|
|
end
|
|
|
|
context "with no assignments" do
|
|
it 'falls back to the default' do
|
|
expect(helper.page_image).to match_asset_path 'assets/gitlab_logo.png'
|
|
end
|
|
end
|
|
end
|
|
end
|
|
|
|
describe 'page_card_attributes' do
|
|
it 'raises ArgumentError when given more than two attributes' do
|
|
map = { foo: 'foo', bar: 'bar', baz: 'baz' }
|
|
|
|
expect { helper.page_card_attributes(map) }
|
|
.to raise_error(ArgumentError, /more than two attributes/)
|
|
end
|
|
|
|
it 'rejects blank values' do
|
|
map = { foo: 'foo', bar: '' }
|
|
helper.page_card_attributes(map)
|
|
|
|
expect(helper.page_card_attributes).to eq({ foo: 'foo' })
|
|
end
|
|
end
|
|
|
|
describe 'page_card_meta_tags' do
|
|
it 'returns the twitter:label and twitter:data tags' do
|
|
allow(helper).to receive(:page_card_attributes).and_return(foo: 'bar')
|
|
|
|
tags = helper.page_card_meta_tags
|
|
|
|
aggregate_failures do
|
|
expect(tags).to include %q(<meta property="twitter:label1" content="foo" />)
|
|
expect(tags).to include %q(<meta property="twitter:data1" content="bar" />)
|
|
end
|
|
end
|
|
|
|
it 'escapes content' do
|
|
allow(helper).to receive(:page_card_attributes)
|
|
.and_return(foo: %q{foo" http-equiv="refresh}.html_safe)
|
|
|
|
tags = helper.page_card_meta_tags
|
|
|
|
expect(tags).to include(%q{content="foo" http-equiv="refresh"})
|
|
end
|
|
end
|
|
end
|