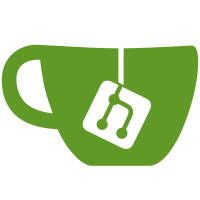
Detect if pipeline runs for a GitHub pull request When using a mirror for CI/CD only we register a pull_request webhook. When a pull_request webhook is received, if the source branch SHA matches the actual head of the branch in the repository we create immediately a new pipeline for the external pull request. Otherwise we store the pull request info for when the push webhook is received. When using "only/except: external_pull_requests" we can detect if the pipeline has a open pull request on GitHub and create or not the job based on that.
29 lines
824 B
Ruby
29 lines
824 B
Ruby
# frozen_string_literal: true
|
|
|
|
# This service is responsible for creating a pipeline for a given
|
|
# ExternalPullRequest coming from other providers such as GitHub.
|
|
|
|
module ExternalPullRequests
|
|
class CreatePipelineService < BaseService
|
|
def execute(pull_request)
|
|
return unless pull_request.open? && pull_request.actual_branch_head?
|
|
|
|
create_pipeline_for(pull_request)
|
|
end
|
|
|
|
private
|
|
|
|
def create_pipeline_for(pull_request)
|
|
Ci::CreatePipelineService.new(project, current_user, create_params(pull_request))
|
|
.execute(:external_pull_request_event, external_pull_request: pull_request)
|
|
end
|
|
|
|
def create_params(pull_request)
|
|
{
|
|
ref: pull_request.source_ref,
|
|
source_sha: pull_request.source_sha,
|
|
target_sha: pull_request.target_sha
|
|
}
|
|
end
|
|
end
|
|
end
|