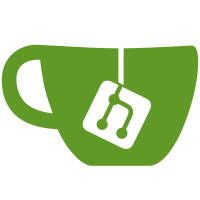
Part of adding Let's Encrypt certificates for pages domains Add acme-client gem Client is being initialized by private key stored in secrets.yml Let's Encrypt account is being created lazily. If it's already created, Acme::Client just gets account_kid by calling new_account method Make Let's Encrypt client an instance Wrap order and challenge classes
154 lines
4 KiB
Ruby
154 lines
4 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
class Admin::ApplicationSettingsController < Admin::ApplicationController
|
|
include InternalRedirect
|
|
before_action :set_application_setting
|
|
|
|
def show
|
|
end
|
|
|
|
def integrations
|
|
end
|
|
|
|
def repository
|
|
end
|
|
|
|
def templates
|
|
end
|
|
|
|
def ci_cd
|
|
end
|
|
|
|
def reporting
|
|
end
|
|
|
|
def metrics_and_profiling
|
|
end
|
|
|
|
def network
|
|
end
|
|
|
|
def geo
|
|
end
|
|
|
|
def preferences
|
|
end
|
|
|
|
def update
|
|
successful = ApplicationSettings::UpdateService
|
|
.new(@application_setting, current_user, application_setting_params)
|
|
.execute
|
|
|
|
if recheck_user_consent?
|
|
session[:ask_for_usage_stats_consent] = current_user.requires_usage_stats_consent?
|
|
end
|
|
|
|
redirect_path = referer_path(request) || admin_application_settings_path
|
|
|
|
respond_to do |format|
|
|
if successful
|
|
format.json { head :ok }
|
|
format.html { redirect_to redirect_path, notice: _('Application settings saved successfully') }
|
|
else
|
|
format.json { head :bad_request }
|
|
format.html { render :show }
|
|
end
|
|
end
|
|
end
|
|
|
|
def usage_data
|
|
respond_to do |format|
|
|
format.html do
|
|
usage_data_json = JSON.pretty_generate(Gitlab::UsageData.data)
|
|
|
|
render html: Gitlab::Highlight.highlight('payload.json', usage_data_json, language: 'json')
|
|
end
|
|
format.json { render json: Gitlab::UsageData.to_json }
|
|
end
|
|
end
|
|
|
|
def reset_registration_token
|
|
@application_setting.reset_runners_registration_token!
|
|
|
|
flash[:notice] = _('New runners registration token has been generated!')
|
|
redirect_to admin_runners_path
|
|
end
|
|
|
|
def reset_health_check_token
|
|
@application_setting.reset_health_check_access_token!
|
|
flash[:notice] = _('New health check access token has been generated!')
|
|
redirect_back_or_default
|
|
end
|
|
|
|
def clear_repository_check_states
|
|
RepositoryCheck::ClearWorker.perform_async
|
|
|
|
redirect_to(
|
|
admin_application_settings_path,
|
|
notice: _('Started asynchronous removal of all repository check states.')
|
|
)
|
|
end
|
|
|
|
# Getting ToS url requires `directory` api call to Let's Encrypt
|
|
# which could result in 500 error/slow rendering on settings page
|
|
# Because of that we use separate controller action
|
|
def lets_encrypt_terms_of_service
|
|
redirect_to ::Gitlab::LetsEncrypt.terms_of_service_url
|
|
end
|
|
|
|
private
|
|
|
|
def set_application_setting
|
|
@application_setting = Gitlab::CurrentSettings.current_application_settings
|
|
end
|
|
|
|
def application_setting_params
|
|
params[:application_setting] ||= {}
|
|
|
|
if params[:application_setting].key?(:enabled_oauth_sign_in_sources)
|
|
enabled_oauth_sign_in_sources = params[:application_setting].delete(:enabled_oauth_sign_in_sources)
|
|
enabled_oauth_sign_in_sources&.delete("")
|
|
|
|
params[:application_setting][:disabled_oauth_sign_in_sources] =
|
|
AuthHelper.button_based_providers.map(&:to_s) -
|
|
Array(enabled_oauth_sign_in_sources)
|
|
end
|
|
|
|
params[:application_setting][:import_sources]&.delete("")
|
|
params[:application_setting][:restricted_visibility_levels]&.delete("")
|
|
params.delete(:domain_blacklist_raw) if params[:domain_blacklist_file]
|
|
|
|
params.require(:application_setting).permit(
|
|
visible_application_setting_attributes
|
|
)
|
|
end
|
|
|
|
def recheck_user_consent?
|
|
return false unless session[:ask_for_usage_stats_consent]
|
|
return false unless params[:application_setting]
|
|
|
|
params[:application_setting].key?(:usage_ping_enabled) || params[:application_setting].key?(:version_check_enabled)
|
|
end
|
|
|
|
def visible_application_setting_attributes
|
|
[
|
|
*::ApplicationSettingsHelper.visible_attributes,
|
|
*::ApplicationSettingsHelper.external_authorization_service_attributes,
|
|
*lets_encrypt_visible_attributes,
|
|
:domain_blacklist_file,
|
|
disabled_oauth_sign_in_sources: [],
|
|
import_sources: [],
|
|
repository_storages: [],
|
|
restricted_visibility_levels: []
|
|
]
|
|
end
|
|
|
|
def lets_encrypt_visible_attributes
|
|
return [] unless Feature.enabled?(:pages_auto_ssl)
|
|
|
|
[
|
|
:lets_encrypt_notification_email,
|
|
:lets_encrypt_terms_of_service_accepted
|
|
]
|
|
end
|
|
end
|