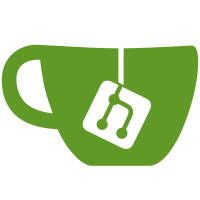
There is a race condition in DestroyGroupService now that projects are deleted asynchronously: 1. User attempts to delete group 2. DestroyGroupService iterates through all projects and schedules a Sidekiq job to delete each Project 3. DestroyGroupService destroys the Group, leaving all its projects without a namespace 4. Projects::DestroyService runs later but the can?(current_user, :remove_project) is `false` because the user no longer has permission to destroy projects with no namespace. 5. This leaves the project in pending_delete state with no namespace/group. Projects without a namespace or group also adds another problem: it's not possible to destroy the container registry tags, since container_registry_path_with_namespace is the wrong value. The fix is to destroy the group asynchronously and to run execute directly on Projects::DestroyService. Closes #17893
60 lines
2 KiB
Ruby
60 lines
2 KiB
Ruby
require 'spec_helper'
|
|
|
|
describe DeleteUserService, services: true do
|
|
describe "Deletes a user and all their personal projects" do
|
|
let!(:user) { create(:user) }
|
|
let!(:current_user) { create(:user) }
|
|
let!(:namespace) { create(:namespace, owner: user) }
|
|
let!(:project) { create(:project, namespace: namespace) }
|
|
|
|
context 'no options are given' do
|
|
it 'deletes the user' do
|
|
user_data = DeleteUserService.new(current_user).execute(user)
|
|
|
|
expect { user_data['email'].to eq(user.email) }
|
|
expect { User.find(user.id) }.to raise_error(ActiveRecord::RecordNotFound)
|
|
expect { Namespace.with_deleted.find(user.namespace.id) }.to raise_error(ActiveRecord::RecordNotFound)
|
|
end
|
|
|
|
it 'will delete the project in the near future' do
|
|
expect_any_instance_of(Projects::DestroyService).to receive(:async_execute).once
|
|
|
|
DeleteUserService.new(current_user).execute(user)
|
|
end
|
|
end
|
|
|
|
context "solo owned groups present" do
|
|
let(:solo_owned) { create(:group) }
|
|
let(:member) { create(:group_member) }
|
|
let(:user) { member.user }
|
|
|
|
before do
|
|
solo_owned.group_members = [member]
|
|
DeleteUserService.new(current_user).execute(user)
|
|
end
|
|
|
|
it 'does not delete the user' do
|
|
expect(User.find(user.id)).to eq user
|
|
end
|
|
end
|
|
|
|
context "deletions with solo owned groups" do
|
|
let(:solo_owned) { create(:group) }
|
|
let(:member) { create(:group_member) }
|
|
let(:user) { member.user }
|
|
|
|
before do
|
|
solo_owned.group_members = [member]
|
|
DeleteUserService.new(current_user).execute(user, delete_solo_owned_groups: true)
|
|
end
|
|
|
|
it 'deletes solo owned groups' do
|
|
expect { Project.find(solo_owned.id) }.to raise_error(ActiveRecord::RecordNotFound)
|
|
end
|
|
|
|
it 'deletes the user' do
|
|
expect { User.find(user.id) }.to raise_error(ActiveRecord::RecordNotFound)
|
|
end
|
|
end
|
|
end
|
|
end
|