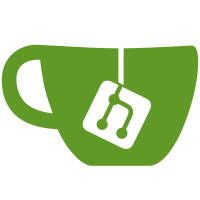
The `access_git` and `access_api` were currently never checked for anonymous users. And they would also be allowed access: An anonymous user can clone and pull from a public repo An anonymous user can request public information from the API So the policy didn't actually reflect what we were enforcing.
68 lines
1.6 KiB
Ruby
68 lines
1.6 KiB
Ruby
class GlobalPolicy < BasePolicy
|
|
desc "User is blocked"
|
|
with_options scope: :user, score: 0
|
|
condition(:blocked) { @user&.blocked? }
|
|
|
|
desc "User is an internal user"
|
|
with_options scope: :user, score: 0
|
|
condition(:internal) { @user&.internal? }
|
|
|
|
desc "User's access has been locked"
|
|
with_options scope: :user, score: 0
|
|
condition(:access_locked) { @user&.access_locked? }
|
|
|
|
condition(:can_create_fork, scope: :user) { @user && @user.manageable_namespaces.any? { |namespace| @user.can?(:create_projects, namespace) } }
|
|
|
|
condition(:required_terms_not_accepted, scope: :user, score: 0) do
|
|
@user&.required_terms_not_accepted?
|
|
end
|
|
|
|
rule { anonymous }.policy do
|
|
prevent :log_in
|
|
prevent :receive_notifications
|
|
prevent :use_quick_actions
|
|
prevent :create_group
|
|
end
|
|
|
|
rule { default }.policy do
|
|
enable :log_in
|
|
enable :access_api
|
|
enable :access_git
|
|
enable :receive_notifications
|
|
enable :use_quick_actions
|
|
end
|
|
|
|
rule { blocked | internal }.policy do
|
|
prevent :log_in
|
|
prevent :access_api
|
|
prevent :access_git
|
|
prevent :receive_notifications
|
|
prevent :use_quick_actions
|
|
end
|
|
|
|
rule { required_terms_not_accepted }.policy do
|
|
prevent :access_api
|
|
prevent :access_git
|
|
end
|
|
|
|
rule { can_create_group }.policy do
|
|
enable :create_group
|
|
end
|
|
|
|
rule { can_create_fork }.policy do
|
|
enable :create_fork
|
|
end
|
|
|
|
rule { access_locked }.policy do
|
|
prevent :log_in
|
|
end
|
|
|
|
rule { ~(anonymous & restricted_public_level) }.policy do
|
|
enable :read_users_list
|
|
end
|
|
|
|
rule { admin }.policy do
|
|
enable :read_custom_attribute
|
|
enable :update_custom_attribute
|
|
end
|
|
end
|